mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 11:17:49 +00:00
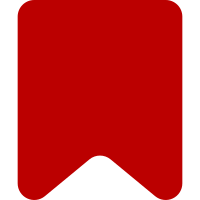
* This patch needs intensive testing on Redis delayed job queue * This patch is -2 mainly for redis/phpredis are not ready on test/test2/mediawiki To test this locally, you need to: * set up Redis and phpredis locally * add the following to localSettings.php $wgJobTypeConf['MWEchoNotificationEmailBundleJob'] = array( 'class' => 'JobQueueRedis', 'redisServer' => '127.0.0.1', 'redisConfig' => array( 'connectTimeout' => 1 ), 'claimTTL' => 3600, 'checkDelay' => true ); * set $wgMainCacheType to CACHE_DB or memcache * set $wgEchoBundleEmailInterval to smaller number for testing purpose, 0 to disable email bundling Change-Id: I9313e7f6ed3e13478cec294b5b8408fe8e941faf
52 lines
1.1 KiB
PHP
52 lines
1.1 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Email Bundling for database storage
|
|
*/
|
|
class MWDbEchoEmailBundler extends MWEchoEmailBundler {
|
|
|
|
/**
|
|
* Retrieve the base event for email bundling, the one with the largest eeb_id
|
|
* @return bool
|
|
*/
|
|
protected function retrieveBaseEvent() {
|
|
$dbr = MWEchoDbFactory::getDB( DB_SLAVE );
|
|
$res = $dbr->selectRow(
|
|
array( 'echo_email_batch' ),
|
|
array( 'eeb_event_id' ),
|
|
array(
|
|
'eeb_user_id' => $this->mUser->getId(),
|
|
'eeb_event_hash' => $this->bundleHash
|
|
),
|
|
__METHOD__,
|
|
array( 'ORDER BY' => 'eeb_event_priority DESC, eeb_id DESC', 'LIMIT' => 1 )
|
|
);
|
|
if ( !$res ) {
|
|
return false;
|
|
}
|
|
$this->baseEvent = EchoEvent::newFromId( $res->eeb_event_id );
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* Clear processed events from the queue
|
|
*/
|
|
protected function clearProcessedEvent() {
|
|
if ( !$this->baseEvent ) {
|
|
return;
|
|
}
|
|
$conds = array( 'eeb_user_id' => $this->mUser->getId(), 'eeb_event_hash' => $this->bundleHash );
|
|
|
|
$conds[] = 'eeb_event_id <= ' . intval( $this->baseEvent->getId() );
|
|
|
|
$dbw = MWEchoDbFactory::getDB( DB_MASTER );
|
|
$dbw->delete(
|
|
'echo_email_batch',
|
|
$conds,
|
|
__METHOD__,
|
|
array()
|
|
);
|
|
}
|
|
|
|
}
|