mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-23 10:22:05 +00:00
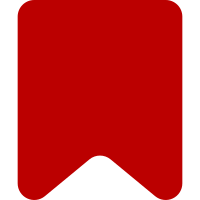
Changes to the use statements done automatically via script Addition of missing use statement done manually Change-Id: I3d7a1ffe167b69d3f4ce51d0c248c758e1cdd70c
63 lines
1.7 KiB
PHP
63 lines
1.7 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Context\DerivativeContext;
|
|
use MediaWiki\Context\RequestContext;
|
|
use MediaWiki\Extension\Notifications\Mapper\NotificationMapper;
|
|
use MediaWiki\Extension\Notifications\Model\Event;
|
|
use MediaWiki\User\User;
|
|
use MediaWiki\User\UserIdentity;
|
|
|
|
/**
|
|
* Tests for the built in notification types
|
|
*
|
|
* @group Database
|
|
*/
|
|
class NotificationsTest extends MediaWikiIntegrationTestCase {
|
|
|
|
/** @var User */
|
|
private $sysop;
|
|
|
|
protected function setUp(): void {
|
|
parent::setUp();
|
|
$this->sysop = $this->getTestSysop()->getUser();
|
|
}
|
|
|
|
/**
|
|
* Helper function to get a user's latest notification
|
|
* @param UserIdentity $user
|
|
* @return Event
|
|
*/
|
|
public static function getLatestNotification( $user ) {
|
|
$notifMapper = new NotificationMapper();
|
|
$notifs = $notifMapper->fetchUnreadByUser( $user, 1, '', [ 'user-rights' ] );
|
|
$notif = array_pop( $notifs );
|
|
|
|
return $notif->getEvent();
|
|
}
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Notifications\Hooks::onUserGroupsChanged
|
|
*/
|
|
public function testUserRightsNotif() {
|
|
$user = new User();
|
|
$user->setName( 'Dummy' );
|
|
$user->addToDatabase();
|
|
|
|
$context = new DerivativeContext( RequestContext::getMain() );
|
|
$context->setUser( $this->sysop );
|
|
$ur = $this->getServiceContainer()
|
|
->getSpecialPageFactory()
|
|
->getPage( 'Userrights' );
|
|
$ur->setContext( $context );
|
|
$ur->doSaveUserGroups( $user, [ 'sysop' ], [], 'reason' );
|
|
$event = self::getLatestNotification( $user );
|
|
$this->assertEquals( 'user-rights', $event->getType() );
|
|
$this->assertEquals( $this->sysop->getName(), $event->getAgent()->getName() );
|
|
$extra = $event->getExtra();
|
|
$this->assertArrayHasKey( 'add', $extra );
|
|
$this->assertArrayEquals( [ 'sysop' ], $extra['add'] );
|
|
$this->assertArrayEquals( [], $extra['remove'] );
|
|
}
|
|
|
|
}
|