mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-24 02:48:18 +00:00
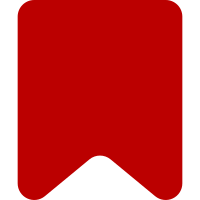
These types can be passed a parameters to any file without creating a dependency, so it makes more sense to allow the globally. Change-Id: I5504465fd997b46547642e7046993b370b85586e
48 lines
1.4 KiB
JavaScript
48 lines
1.4 KiB
JavaScript
var ThreadItem = require( './ThreadItem.js' );
|
|
|
|
/**
|
|
* A comment item
|
|
*
|
|
* @class CommentItem
|
|
* @extends ThreadItem
|
|
* @constructor
|
|
* @param {number} level
|
|
* @param {Object} range
|
|
* @param {Object[]} [signatureRanges] Objects describing the extent of signatures (plus
|
|
* timestamps) for this comment. There is always at least one signature, but there may be
|
|
* multiple. The author and timestamp of the comment is determined from the first signature.
|
|
* The last node in every signature range is a node containing the timestamp.
|
|
* @param {moment} [timestamp] Timestamp (Moment object)
|
|
* @param {string} [author] Comment author's username
|
|
*/
|
|
function CommentItem( level, range, signatureRanges, timestamp, author ) {
|
|
// Parent constructor
|
|
CommentItem.super.call( this, 'comment', level, range );
|
|
|
|
this.signatureRanges = signatureRanges || [];
|
|
this.timestamp = timestamp || null;
|
|
this.author = author || null;
|
|
|
|
/**
|
|
* @member {ThreadItem} Parent thread item
|
|
*/
|
|
this.parent = null;
|
|
}
|
|
|
|
OO.inheritClass( CommentItem, ThreadItem );
|
|
|
|
/**
|
|
* @return {HeadingItem} Closest ancestor which is a HeadingItem
|
|
*/
|
|
CommentItem.prototype.getHeading = function () {
|
|
var parent = this;
|
|
while ( parent && parent.type !== 'heading' ) {
|
|
parent = parent.parent;
|
|
}
|
|
return parent;
|
|
};
|
|
|
|
// TODO: Implement getBodyRange/getBodyHTML/getBodyText/getMentions if required
|
|
|
|
module.exports = CommentItem;
|