mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-23 18:38:18 +00:00
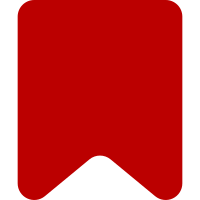
MediaWiki's PHPCS plugin requires documentation comments on all properties, unless those properties are typed. This has potential to introduce bugs – in particular, because typed properties without a default value will throw an exception if their value is accessed before it's defined, while previously they defaulted to null. I fixed this when I found it (making them nullable and null by default), but I may have missed some cases. Change-Id: If5b1f4d542ce3e1b69327ee4283f7c3e133a62a0
101 lines
2.2 KiB
PHP
101 lines
2.2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools;
|
|
|
|
use MediaWiki\Linker\LinkTarget;
|
|
use MediaWiki\User\UserIdentity;
|
|
|
|
/**
|
|
* Representation of a subscription to a given topic.
|
|
*/
|
|
class SubscriptionItem {
|
|
private UserIdentity $user;
|
|
private string $itemName;
|
|
private LinkTarget $linkTarget;
|
|
private int $state;
|
|
private ?string $createdTimestamp;
|
|
private ?string $notifiedTimestamp;
|
|
|
|
/**
|
|
* @param UserIdentity $user
|
|
* @param string $itemName
|
|
* @param LinkTarget $linkTarget
|
|
* @param int $state One of SubscriptionStore::STATE_* constants
|
|
* @param string|null $createdTimestamp When the subscription was created
|
|
* @param string|null $notifiedTimestamp When the item subscribed to last tried to trigger
|
|
* a notification (even if muted).
|
|
*/
|
|
public function __construct(
|
|
UserIdentity $user,
|
|
string $itemName,
|
|
LinkTarget $linkTarget,
|
|
int $state,
|
|
?string $createdTimestamp,
|
|
?string $notifiedTimestamp
|
|
) {
|
|
$this->user = $user;
|
|
$this->itemName = $itemName;
|
|
$this->linkTarget = $linkTarget;
|
|
$this->state = $state;
|
|
$this->createdTimestamp = $createdTimestamp;
|
|
$this->notifiedTimestamp = $notifiedTimestamp;
|
|
}
|
|
|
|
/**
|
|
* @return UserIdentity
|
|
*/
|
|
public function getUserIdentity(): UserIdentity {
|
|
return $this->user;
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getItemName(): string {
|
|
return $this->itemName;
|
|
}
|
|
|
|
/**
|
|
* @return LinkTarget
|
|
*/
|
|
public function getLinkTarget(): LinkTarget {
|
|
return $this->linkTarget;
|
|
}
|
|
|
|
/**
|
|
* Get the creation timestamp of this entry.
|
|
*
|
|
* @return string|null
|
|
*/
|
|
public function getCreatedTimestamp(): ?string {
|
|
return $this->createdTimestamp;
|
|
}
|
|
|
|
/**
|
|
* Get the notification timestamp of this entry.
|
|
*
|
|
* @return string|null
|
|
*/
|
|
public function getNotificationTimestamp(): ?string {
|
|
return $this->notifiedTimestamp;
|
|
}
|
|
|
|
/**
|
|
* Get the subscription status of this entry.
|
|
*
|
|
* @return int One of SubscriptionStore::STATE_* constants
|
|
*/
|
|
public function getState(): int {
|
|
return $this->state;
|
|
}
|
|
|
|
/**
|
|
* Check if the notification is muted
|
|
*
|
|
* @return bool
|
|
*/
|
|
public function isMuted(): bool {
|
|
return $this->state === SubscriptionStore::STATE_UNSUBSCRIBED;
|
|
}
|
|
}
|