mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-24 19:08:16 +00:00
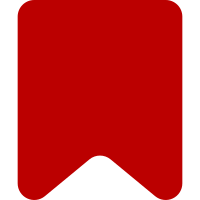
This is similar to the code we already have in JS tests, but instead of printing to the console where you have to copy-paste from, it just overwrites the files. Also, update all of the expected results by this method. Changes in the expected outputs: * In JSON files, the "warnings" are now always in the same place regardless of the type of the warning. * In all HTML files, self-closing tags now include the trailing slash, some characters are no longer encoded as entities when not necessary, and attributes may be single-quoted when that makes them shorter. * In Parsoid HTML files, the header is no longer terribly mangled. Other notes: * CommentParserTest.php: Change the output of serializeComments() to be in similar order as in JS, to reduce the diffs in this commit and because it's a better order for humans. * modifier.test.js: Remove some hacks that were working around small inconsistencies between the previous expected outputs and the actual outputs. Change-Id: I9f764640dae823321c0ac35898fa4db03f1ca364
166 lines
4.6 KiB
JavaScript
166 lines
4.6 KiB
JavaScript
var
|
|
testUtils = require( './testUtils.js' ),
|
|
Parser = require( 'ext.discussionTools.init' ).Parser,
|
|
modifier = require( 'ext.discussionTools.init' ).modifier;
|
|
|
|
QUnit.module( 'mw.dt.modifier', testUtils.newEnvironment() );
|
|
|
|
QUnit.test( '#addListItem/#removeAddedListItem', function ( assert ) {
|
|
var cases = require( '../cases/modified.json' ),
|
|
fixture = document.getElementById( 'qunit-fixture' );
|
|
|
|
cases.forEach( function ( caseItem ) {
|
|
var actualHtml, expectedHtml, reverseActualHtml, reverseExpectedHtml,
|
|
i, comments, nodes, node, parser,
|
|
dom = mw.template.get( 'test.DiscussionTools', caseItem.dom ).render(),
|
|
expected = mw.template.get( 'test.DiscussionTools', caseItem.expected ).render(),
|
|
config = require( caseItem.config ),
|
|
data = require( caseItem.data );
|
|
|
|
testUtils.overrideMwConfig( config );
|
|
testUtils.overrideParserData( data );
|
|
|
|
$( fixture ).empty().append( expected );
|
|
expectedHtml = fixture.innerHTML;
|
|
|
|
$( fixture ).empty().append( dom );
|
|
reverseExpectedHtml = fixture.innerHTML;
|
|
|
|
parser = new Parser( fixture );
|
|
comments = parser.getCommentItems();
|
|
|
|
// Add a reply to every comment. Note that this inserts *all* of the replies, unlike the real
|
|
// thing, which only deals with one at a time. This isn't ideal but resetting everything after
|
|
// every reply would be super slow.
|
|
nodes = [];
|
|
for ( i = 0; i < comments.length; i++ ) {
|
|
node = modifier.addListItem( comments[ i ] );
|
|
node.textContent = 'Reply to ' + comments[ i ].id;
|
|
nodes.push( node );
|
|
}
|
|
|
|
// Uncomment this to get updated content for the "modified HTML" files, for copy/paste:
|
|
// console.log( fixture.innerHTML );
|
|
|
|
actualHtml = fixture.innerHTML;
|
|
|
|
assert.strictEqual(
|
|
actualHtml,
|
|
expectedHtml,
|
|
caseItem.name
|
|
);
|
|
|
|
// Now discard the replies and verify we get the original document back.
|
|
for ( i = 0; i < nodes.length; i++ ) {
|
|
modifier.removeAddedListItem( nodes[ i ] );
|
|
}
|
|
|
|
reverseActualHtml = fixture.innerHTML;
|
|
assert.strictEqual(
|
|
reverseActualHtml,
|
|
reverseExpectedHtml,
|
|
caseItem.name + ' (discard replies)'
|
|
);
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( '#addReplyLink', function ( assert ) {
|
|
var cases = require( '../cases/reply.json' ),
|
|
fixture = document.getElementById( 'qunit-fixture' );
|
|
|
|
cases.forEach( function ( caseItem ) {
|
|
var actualHtml, expectedHtml,
|
|
i, comments, linkNode, parser,
|
|
dom = mw.template.get( 'test.DiscussionTools', caseItem.dom ).render(),
|
|
expected = mw.template.get( 'test.DiscussionTools', caseItem.expected ).render(),
|
|
config = require( caseItem.config ),
|
|
data = require( caseItem.data );
|
|
|
|
testUtils.overrideMwConfig( config );
|
|
testUtils.overrideParserData( data );
|
|
|
|
$( fixture ).empty().append( expected );
|
|
expectedHtml = fixture.innerHTML;
|
|
|
|
$( fixture ).empty().append( dom );
|
|
|
|
parser = new Parser( fixture );
|
|
comments = parser.getCommentItems();
|
|
|
|
// Add a reply link to every comment.
|
|
for ( i = 0; i < comments.length; i++ ) {
|
|
linkNode = document.createElement( 'a' );
|
|
linkNode.textContent = 'Reply';
|
|
linkNode.href = '#';
|
|
modifier.addReplyLink( comments[ i ], linkNode );
|
|
}
|
|
|
|
// Uncomment this to get updated content for the "reply HTML" files, for copy/paste:
|
|
// console.log( fixture.innerHTML );
|
|
|
|
actualHtml = fixture.innerHTML;
|
|
|
|
assert.strictEqual(
|
|
actualHtml,
|
|
expectedHtml,
|
|
caseItem.name
|
|
);
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( '#unwrapList', function ( assert ) {
|
|
var cases = require( '../cases/unwrap.json' );
|
|
|
|
cases.forEach( function ( caseItem ) {
|
|
var container = document.createElement( 'div' );
|
|
|
|
container.innerHTML = caseItem.html;
|
|
modifier.unwrapList( container.childNodes[ caseItem.index || 0 ] );
|
|
|
|
assert.strictEqual(
|
|
container.innerHTML,
|
|
caseItem.expected,
|
|
caseItem.name
|
|
);
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'isWikitextSigned', function ( assert ) {
|
|
var cases = require( '../cases/isWikitextSigned.json' );
|
|
|
|
cases.forEach( function ( caseItem ) {
|
|
assert.strictEqual(
|
|
modifier.isWikitextSigned( caseItem.wikitext ),
|
|
caseItem.expected,
|
|
caseItem.msg
|
|
);
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'isHtmlSigned', function ( assert ) {
|
|
var cases = require( '../cases/isHtmlSigned.json' );
|
|
|
|
cases.forEach( function ( caseItem ) {
|
|
var container = document.createElement( 'div' );
|
|
container.innerHTML = caseItem.html;
|
|
|
|
assert.strictEqual(
|
|
modifier.isHtmlSigned( container ),
|
|
caseItem.expected,
|
|
caseItem.msg
|
|
);
|
|
} );
|
|
} );
|
|
|
|
QUnit.test( 'sanitizeWikitextLinebreaks', function ( assert ) {
|
|
var cases = require( '../cases/sanitize-wikitext-linebreaks.json' );
|
|
|
|
cases.forEach( function ( caseItem ) {
|
|
assert.strictEqual(
|
|
modifier.sanitizeWikitextLinebreaks( caseItem.wikitext ),
|
|
caseItem.expected,
|
|
caseItem.msg
|
|
);
|
|
} );
|
|
} );
|