mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-24 08:23:52 +00:00
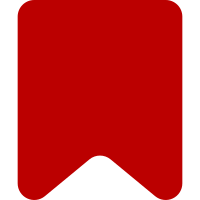
composer: * mediawiki/mediawiki-codesniffer: 36.0.0 → 37.0.0 npm: * postcss: 7.0.35 → 7.0.36 * https://npmjs.com/advisories/1693 (CVE-2021-23368) * glob-parent: 5.1.1 → 5.1.2 * https://npmjs.com/advisories/1751 (CVE-2020-28469) * trim-newlines: 3.0.0 → 3.0.1 * https://npmjs.com/advisories/1753 (CVE-2021-33623) Change-Id: I7a71e23da561599da417db3b3077b78d91173bbc
94 lines
2.4 KiB
PHP
94 lines
2.4 KiB
PHP
<?php
|
|
/**
|
|
* DiscussionTools echo hooks
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @license MIT
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools\Hooks;
|
|
|
|
use EchoEvent;
|
|
use MediaWiki\Extension\DiscussionTools\Notifications\EventDispatcher;
|
|
use MediaWiki\MediaWikiServices;
|
|
use MediaWiki\Revision\RevisionRecord;
|
|
|
|
class EchoHooks {
|
|
/**
|
|
* Add notification events to Echo
|
|
*
|
|
* @param array &$notifications
|
|
* @param array &$notificationCategories
|
|
* @param array &$icons
|
|
*/
|
|
public static function onBeforeCreateEchoEvent(
|
|
array &$notifications,
|
|
array &$notificationCategories,
|
|
array &$icons
|
|
) {
|
|
$services = MediaWikiServices::getInstance();
|
|
$dtConfig = $services->getConfigFactory()->makeConfig( 'discussiontools' );
|
|
if ( !$dtConfig->get( 'DiscussionToolsEnableTopicSubscriptionBackend' ) ) {
|
|
// Topic subscriptions not available on wiki.
|
|
return;
|
|
}
|
|
|
|
$notificationCategories['dt-subscription'] = [
|
|
'priority' => 3,
|
|
'tooltip' => 'echo-pref-tooltip-dt-subscription',
|
|
];
|
|
|
|
$notifications['dt-subscribed-new-comment'] = [
|
|
'category' => 'dt-subscription',
|
|
'group' => 'interactive',
|
|
'section' => 'message',
|
|
'user-locators' => [
|
|
'MediaWiki\\Extension\\DiscussionTools\\Notifications\\EventDispatcher::locateSubscribedUsers'
|
|
],
|
|
// Exclude mentioned users and talk page owner from our notification, to avoid
|
|
// duplicate notifications for a single comment
|
|
'user-filters' => [
|
|
[
|
|
"EchoUserLocator::locateFromEventExtra",
|
|
[ "mentioned-users" ]
|
|
],
|
|
"EchoUserLocator::locateTalkPageOwner"
|
|
],
|
|
'presentation-model' =>
|
|
'MediaWiki\\Extension\\DiscussionTools\\Notifications\\SubscribedNewCommentPresentationModel',
|
|
'bundle' => [
|
|
'web' => true,
|
|
'email' => true,
|
|
'expandable' => true,
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @param EchoEvent $event
|
|
* @param string &$bundleString
|
|
* @return bool
|
|
*/
|
|
public static function onEchoGetBundleRules( EchoEvent $event, string &$bundleString ): bool {
|
|
switch ( $event->getType() ) {
|
|
case 'dt-subscribed-new-comment':
|
|
$bundleString = $event->getType() . '-' . $event->getExtraParam( 'subscribed-comment-name' );
|
|
break;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @param array &$events
|
|
* @param RevisionRecord $revision
|
|
* @param bool $isRevert
|
|
*/
|
|
public static function onEchoGetEventsForRevision( array &$events, RevisionRecord $revision, bool $isRevert ) {
|
|
if ( $isRevert ) {
|
|
return;
|
|
}
|
|
EventDispatcher::generateEventsForRevision( $events, $revision );
|
|
}
|
|
}
|