mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-24 10:58:20 +00:00
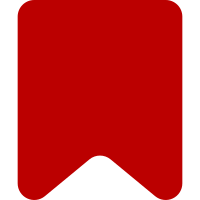
Change code to match the documented consensus formed on T321683: https://www.mediawiki.org/wiki/Manual:Coding_conventions/PHP#Exception_handling * Do not directly throw Exception, Error or MWException * Document checked exceptions with @throws * Do not document unchecked exceptions For this extension, I think it makes sense to consider DOMException an unchecked exception too (in addition to the usual LogicException and RuntimeException). Depends-On: Id07e301c3f20afa135e5469ee234a27354485652 Depends-On: I869af06896b9757af18488b916211c5a41a8c563 Depends-On: I42d9b7465d1406a22ef1b3f6d8de426c60c90e2c Change-Id: Ic9d9efd031a87fa5a93143f714f0adb20f0dd956
187 lines
5 KiB
PHP
187 lines
5 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools;
|
|
|
|
use ApiBase;
|
|
use ApiMain;
|
|
use ApiUsageException;
|
|
use MediaWiki\Extension\DiscussionTools\Hooks\HookUtils;
|
|
use MediaWiki\Extension\VisualEditor\VisualEditorParsoidClientFactory;
|
|
use MediaWiki\Revision\RevisionLookup;
|
|
use MediaWiki\Revision\RevisionRecord;
|
|
use Title;
|
|
use Wikimedia\ParamValidator\ParamValidator;
|
|
|
|
class ApiDiscussionToolsCompare extends ApiBase {
|
|
|
|
private CommentParser $commentParser;
|
|
private VisualEditorParsoidClientFactory $parsoidClientFactory;
|
|
private RevisionLookup $revisionLookup;
|
|
|
|
public function __construct(
|
|
ApiMain $main,
|
|
string $name,
|
|
VisualEditorParsoidClientFactory $parsoidClientFactory,
|
|
CommentParser $commentParser,
|
|
RevisionLookup $revisionLookup
|
|
) {
|
|
parent::__construct( $main, $name );
|
|
$this->parsoidClientFactory = $parsoidClientFactory;
|
|
$this->commentParser = $commentParser;
|
|
$this->revisionLookup = $revisionLookup;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @throws ApiUsageException
|
|
*/
|
|
public function execute() {
|
|
$params = $this->extractRequestParams();
|
|
|
|
$this->requireOnlyOneParameter( $params, 'fromtitle', 'fromrev' );
|
|
$this->requireOnlyOneParameter( $params, 'totitle', 'torev' );
|
|
|
|
if ( isset( $params['torev'] ) ) {
|
|
$toRev = $this->revisionLookup->getRevisionById( $params['torev'] );
|
|
if ( !$toRev ) {
|
|
$this->dieWithError( [ 'apierror-nosuchrevid', $params['torev'] ] );
|
|
}
|
|
|
|
} else {
|
|
$toTitle = Title::newFromText( $params['totitle'] );
|
|
if ( !$toTitle ) {
|
|
$this->dieWithError( [ 'apierror-invalidtitle', wfEscapeWikiText( $params['totitle'] ) ] );
|
|
}
|
|
$toRev = $this->revisionLookup->getRevisionByTitle( $toTitle );
|
|
if ( !$toRev ) {
|
|
$this->dieWithError(
|
|
[ 'apierror-missingrev-title', wfEscapeWikiText( $toTitle->getPrefixedText() ) ],
|
|
'nosuchrevid'
|
|
);
|
|
}
|
|
}
|
|
|
|
// When polling for new comments this is an important optimisation,
|
|
// as usually there is no new revision.
|
|
if ( $toRev->getId() === $params['fromrev'] ) {
|
|
$this->addResult( $toRev, $toRev );
|
|
return;
|
|
}
|
|
|
|
if ( isset( $params['fromrev'] ) ) {
|
|
$fromRev = $this->revisionLookup->getRevisionById( $params['fromrev'] );
|
|
if ( !$fromRev ) {
|
|
$this->dieWithError( [ 'apierror-nosuchrevid', $params['fromrev'] ] );
|
|
}
|
|
|
|
} else {
|
|
$fromTitle = Title::newFromText( $params['fromtitle'] );
|
|
if ( !$fromTitle ) {
|
|
$this->dieWithError( [ 'apierror-invalidtitle', wfEscapeWikiText( $params['fromtitle'] ) ] );
|
|
}
|
|
$fromRev = $this->revisionLookup->getRevisionByTitle( $fromTitle );
|
|
if ( !$fromRev ) {
|
|
$this->dieWithError(
|
|
[ 'apierror-missingrev-title', wfEscapeWikiText( $fromTitle->getPrefixedText() ) ],
|
|
'nosuchrevid'
|
|
);
|
|
}
|
|
}
|
|
|
|
if ( $fromRev->hasSameContent( $toRev ) ) {
|
|
$this->addResult( $fromRev, $toRev );
|
|
return;
|
|
}
|
|
|
|
$fromItemSet = HookUtils::parseRevisionParsoidHtml( $fromRev, __METHOD__ );
|
|
$toItemSet = HookUtils::parseRevisionParsoidHtml( $toRev, __METHOD__ );
|
|
|
|
$removedComments = [];
|
|
foreach ( $fromItemSet->getCommentItems() as $fromComment ) {
|
|
if ( !$toItemSet->findCommentById( $fromComment->getId() ) ) {
|
|
$removedComments[] = $fromComment->jsonSerializeForDiff();
|
|
}
|
|
}
|
|
|
|
$addedComments = [];
|
|
foreach ( $toItemSet->getCommentItems() as $toComment ) {
|
|
if ( !$fromItemSet->findCommentById( $toComment->getId() ) ) {
|
|
$addedComments[] = $toComment->jsonSerializeForDiff();
|
|
}
|
|
}
|
|
|
|
$this->addResult( $fromRev, $toRev, $removedComments, $addedComments );
|
|
}
|
|
|
|
/**
|
|
* Add the result object from revisions and comment lists
|
|
*
|
|
* @param RevisionRecord $fromRev From revision
|
|
* @param RevisionRecord $toRev To revision
|
|
* @param array $removedComments Removed comments
|
|
* @param array $addedComments Added comments
|
|
*/
|
|
protected function addResult(
|
|
RevisionRecord $fromRev, RevisionRecord $toRev, array $removedComments = [], array $addedComments = []
|
|
) {
|
|
$fromTitle = Title::newFromLinkTarget(
|
|
$fromRev->getPageAsLinkTarget()
|
|
);
|
|
$toTitle = Title::newFromLinkTarget(
|
|
$toRev->getPageAsLinkTarget()
|
|
);
|
|
$result = [
|
|
'fromrevid' => $fromRev->getId(),
|
|
'fromtitle' => $fromTitle->getPrefixedText(),
|
|
'torevid' => $toRev->getId(),
|
|
'totitle' => $toTitle->getPrefixedText(),
|
|
'removedcomments' => $removedComments,
|
|
'addedcomments' => $addedComments,
|
|
];
|
|
$this->getResult()->addValue( null, $this->getModuleName(), $result );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getAllowedParams() {
|
|
return [
|
|
'fromtitle' => [
|
|
ApiBase::PARAM_HELP_MSG => 'apihelp-compare-param-fromtitle',
|
|
],
|
|
'fromrev' => [
|
|
ParamValidator::PARAM_TYPE => 'integer',
|
|
ApiBase::PARAM_HELP_MSG => 'apihelp-compare-param-fromrev',
|
|
],
|
|
'totitle' => [
|
|
ApiBase::PARAM_HELP_MSG => 'apihelp-compare-param-totitle',
|
|
],
|
|
'torev' => [
|
|
ParamValidator::PARAM_TYPE => 'integer',
|
|
ApiBase::PARAM_HELP_MSG => 'apihelp-compare-param-torev',
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function needsToken() {
|
|
return false;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function isInternal() {
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function isWriteMode() {
|
|
return false;
|
|
}
|
|
}
|