mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-15 12:00:51 +00:00
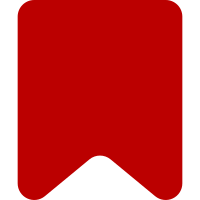
This code is throwing exception for as yet uncertain reasons, which may cause other updates to not be executed (e.g. Echo notifications). Put a try…catch around it while we investigate. Bug: T315383 Change-Id: Ic7aba32369f69c2e8165d5d6d25687a4cb6e0be8
60 lines
1.6 KiB
PHP
60 lines
1.6 KiB
PHP
<?php
|
|
/**
|
|
* DiscussionTools data updates hooks
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @license MIT
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools\Hooks;
|
|
|
|
use DeferrableUpdate;
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItemStore;
|
|
use MediaWiki\Revision\RenderedRevision;
|
|
use MediaWiki\Storage\Hook\RevisionDataUpdatesHook;
|
|
use MWCallableUpdate;
|
|
use MWExceptionHandler;
|
|
use Throwable;
|
|
use Title;
|
|
|
|
class DataUpdatesHooks implements RevisionDataUpdatesHook {
|
|
|
|
/** @var ThreadItemStore */
|
|
private $threadItemStore;
|
|
|
|
/**
|
|
* @param ThreadItemStore $threadItemStore
|
|
*/
|
|
public function __construct(
|
|
ThreadItemStore $threadItemStore
|
|
) {
|
|
$this->threadItemStore = $threadItemStore;
|
|
}
|
|
|
|
/**
|
|
* @param Title $title
|
|
* @param RenderedRevision $renderedRevision
|
|
* @param DeferrableUpdate[] &$updates
|
|
* @return bool|void
|
|
*/
|
|
public function onRevisionDataUpdates( $title, $renderedRevision, &$updates ) {
|
|
// This doesn't trigger on action=purge, only on automatic purge after editing a template or
|
|
// transcluded page, and API action=purge&forcelinkupdate=1.
|
|
|
|
// TODO Deduplicate work between this and the Echo hook (make it use Parsoid too)
|
|
$rev = $renderedRevision->getRevision();
|
|
if ( HookUtils::isAvailableForTitle( $title ) ) {
|
|
$updates[] = new MWCallableUpdate( function () use ( $rev ) {
|
|
try {
|
|
$threadItemSet = HookUtils::parseRevisionParsoidHtml( $rev );
|
|
$this->threadItemStore->insertThreadItems( $rev, $threadItemSet );
|
|
} catch ( Throwable $e ) {
|
|
// Catch errors, so that they don't cause other updates to fail (T315383), but log them.
|
|
MWExceptionHandler::logException( $e );
|
|
}
|
|
}, __METHOD__ );
|
|
}
|
|
}
|
|
}
|