mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-14 19:35:38 +00:00
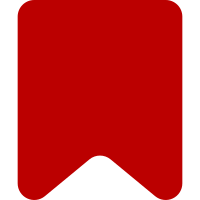
This changes a vast number of test expected-outputs because it affects the order of the keys when serialized. Bug: T315400 Change-Id: I6ad2cded6ba7cb2cc5e5ba37ea60f4b18ecc26be
45 lines
1.5 KiB
PHP
45 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools\ThreadItem;
|
|
|
|
trait HeadingItemTrait {
|
|
// phpcs:disable Squiz.WhiteSpace, MediaWiki.Commenting
|
|
// Required ThreadItem methods (listed for Phan)
|
|
abstract public function getName(): string;
|
|
// Required HeadingItem methods (listed for Phan)
|
|
abstract public function getHeadingLevel(): int;
|
|
abstract public function isPlaceholderHeading(): bool;
|
|
// phpcs:enable
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @suppress PhanTraitParentReference
|
|
*/
|
|
public function jsonSerialize( bool $deep = false, ?callable $callback = null ): array {
|
|
return array_merge( [
|
|
'headingLevel' => $this->isPlaceholderHeading() ? null : $this->getHeadingLevel(),
|
|
// Used for topic subscriptions. Not added to CommentItem's yet as there is
|
|
// no use case for it.
|
|
'name' => $this->getName(),
|
|
], parent::jsonSerialize( $deep, $callback ) );
|
|
}
|
|
|
|
/**
|
|
* Check whether this heading can be used for topic subscriptions.
|
|
*
|
|
* @return bool
|
|
*/
|
|
public function isSubscribable(): bool {
|
|
return (
|
|
// Placeholder headings have nothing to attach the button to.
|
|
!$this->isPlaceholderHeading() &&
|
|
// We only allow subscribing to level 2 headings, because the user interface for sub-headings
|
|
// would be difficult to present.
|
|
$this->getHeadingLevel() === 2 &&
|
|
// Check if the name corresponds to a section that contain no comments (only sub-sections).
|
|
// They can't be distinguished from each other, so disallow subscribing.
|
|
$this->getName() !== 'h-'
|
|
);
|
|
}
|
|
}
|