mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-23 10:29:11 +00:00
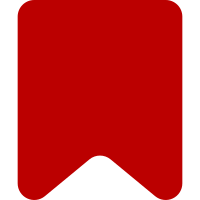
MediaWiki's PHPCS plugin requires documentation comments on all methods, unless those methods are fully typed (all parameters and return value). It turns out that almost all of our methods are fully typed already. Procedure: 1. Find: \*(\s*\*\s*(@param \??[\w\\]+(\|null)? &?\$\w+|@return \??[\w\\]+(\|null)?)\n)+\s*\*/ Replace with: */ This deletes type annotations, except those not representable as PHP type hints such as union types `a|b` or typed arrays `a[]`, or those with documentation beyond type hints, or those on functions with any other annotations. 2. Find: /\*\*/\n\s* Replace with nothing This deletes the remaining comments on methods that had no prose documentation. 3. Undo all changes that PHPCS complains about (those comments were not redundant) 4. Review the diff carefully, these regexps are imprecise :) Change-Id: Ic82e8b23f2996f44951208dbd9cfb4c8e0738dac
86 lines
2 KiB
PHP
86 lines
2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools;
|
|
|
|
use MediaWiki\Linker\LinkTarget;
|
|
use MediaWiki\User\UserIdentity;
|
|
|
|
/**
|
|
* Representation of a subscription to a given topic.
|
|
*/
|
|
class SubscriptionItem {
|
|
private UserIdentity $user;
|
|
private string $itemName;
|
|
private LinkTarget $linkTarget;
|
|
private int $state;
|
|
private ?string $createdTimestamp;
|
|
private ?string $notifiedTimestamp;
|
|
|
|
/**
|
|
* @param UserIdentity $user
|
|
* @param string $itemName
|
|
* @param LinkTarget $linkTarget
|
|
* @param int $state One of SubscriptionStore::STATE_* constants
|
|
* @param string|null $createdTimestamp When the subscription was created
|
|
* @param string|null $notifiedTimestamp When the item subscribed to last tried to trigger
|
|
* a notification (even if muted).
|
|
*/
|
|
public function __construct(
|
|
UserIdentity $user,
|
|
string $itemName,
|
|
LinkTarget $linkTarget,
|
|
int $state,
|
|
?string $createdTimestamp,
|
|
?string $notifiedTimestamp
|
|
) {
|
|
$this->user = $user;
|
|
$this->itemName = $itemName;
|
|
$this->linkTarget = $linkTarget;
|
|
$this->state = $state;
|
|
$this->createdTimestamp = $createdTimestamp;
|
|
$this->notifiedTimestamp = $notifiedTimestamp;
|
|
}
|
|
|
|
public function getUserIdentity(): UserIdentity {
|
|
return $this->user;
|
|
}
|
|
|
|
public function getItemName(): string {
|
|
return $this->itemName;
|
|
}
|
|
|
|
public function getLinkTarget(): LinkTarget {
|
|
return $this->linkTarget;
|
|
}
|
|
|
|
/**
|
|
* Get the creation timestamp of this entry.
|
|
*/
|
|
public function getCreatedTimestamp(): ?string {
|
|
return $this->createdTimestamp;
|
|
}
|
|
|
|
/**
|
|
* Get the notification timestamp of this entry.
|
|
*/
|
|
public function getNotificationTimestamp(): ?string {
|
|
return $this->notifiedTimestamp;
|
|
}
|
|
|
|
/**
|
|
* Get the subscription status of this entry.
|
|
*
|
|
* @return int One of SubscriptionStore::STATE_* constants
|
|
*/
|
|
public function getState(): int {
|
|
return $this->state;
|
|
}
|
|
|
|
/**
|
|
* Check if the notification is muted
|
|
*/
|
|
public function isMuted(): bool {
|
|
return $this->state === SubscriptionStore::STATE_UNSUBSCRIBED;
|
|
}
|
|
}
|