mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-24 19:08:16 +00:00
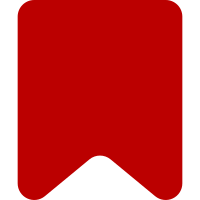
Previously they were added at the end of the text node containing the timestamp, which was usually the end of the line, but not always. And also fix the same problem for inserting the actual replies (or reply widgets). This replaces an undocumented hack that prevented our own reply links from triggering this bug (without it, the reply widget would be inserted before the reply link rather than after). While we're here, remove unintentional spacing that appeared before some reply links, caused by trailing whitespace in text nodes. Add tests for all of the above. Bug: T245695 Change-Id: I354b63e2446bb996176a2e3d76abf944127f307e
182 lines
6.6 KiB
JavaScript
182 lines
6.6 KiB
JavaScript
var
|
|
utils = require( './utils.js' ),
|
|
parser = require( 'ext.discussionTools.parser' ),
|
|
modifier = require( 'ext.discussionTools.modifier' );
|
|
|
|
QUnit.module( 'mw.dt.modifier', utils.newEnvironment() );
|
|
|
|
QUnit.test( '#addListItem/#removeListItem', function ( assert ) {
|
|
var i, j, cases,
|
|
actualHtml, expectedHtml, reverseActualHtml, reverseExpectedHtml,
|
|
comments, nodes, node, fixture;
|
|
|
|
cases = [
|
|
{
|
|
name: 'plwiki oldparser',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/pl-big-oldparser/pl-big-oldparser.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/pl-big-oldparser/pl-big-oldparser-modified.html' ).render(),
|
|
config: require( './data/plwiki-config.json' ),
|
|
data: require( './data/plwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'plwiki parsoid',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/pl-big-parsoid/pl-big-parsoid.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/pl-big-parsoid/pl-big-parsoid-modified.html' ).render(),
|
|
config: require( './data/plwiki-config.json' ),
|
|
data: require( './data/plwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'enwiki oldparser',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/en-big-oldparser/en-big-oldparser.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/en-big-oldparser/en-big-oldparser-modified.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'enwiki parsoid',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/en-big-parsoid/en-big-parsoid.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/en-big-parsoid/en-big-parsoid-modified.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'Must split a list to reply to one of the comments',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/split-list/split-list.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/split-list/split-list-modified.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'Must split a list to reply to one of the comments (version 2)',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/split-list2/split-list2.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/split-list2/split-list2-modified.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'Signatures in funny places',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/signatures-funny/signatures-funny.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/signatures-funny/signatures-funny-modified.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
}
|
|
];
|
|
|
|
fixture = document.getElementById( 'qunit-fixture' );
|
|
|
|
for ( i = 0; i < cases.length; i++ ) {
|
|
utils.overrideMwConfig( cases[ i ].config );
|
|
utils.overrideParserData( cases[ i ].data );
|
|
|
|
$( fixture ).empty().append( cases[ i ].expected );
|
|
expectedHtml = fixture.innerHTML;
|
|
|
|
$( fixture ).empty().append( cases[ i ].dom.clone() );
|
|
reverseExpectedHtml = fixture.innerHTML;
|
|
|
|
comments = parser.getComments( fixture );
|
|
parser.groupThreads( comments );
|
|
|
|
// Add a reply to every comment. Note that this inserts *all* of the replies, unlike the real
|
|
// thing, which only deals with one at a time. This isn't ideal but resetting everything after
|
|
// every reply would be super slow.
|
|
nodes = [];
|
|
for ( j = 0; j < comments.length; j++ ) {
|
|
if ( comments[ j ].type === 'heading' ) {
|
|
continue;
|
|
}
|
|
node = modifier.addListItem( comments[ j ] );
|
|
node.textContent = 'Reply to ' + comments[ j ].id;
|
|
nodes.push( node );
|
|
}
|
|
|
|
// Uncomment this to get updated content for the the "modified HTML" files, for copy/paste:
|
|
// console.log( fixture.innerHTML );
|
|
|
|
actualHtml = fixture.innerHTML.trim();
|
|
|
|
assert.strictEqual(
|
|
actualHtml,
|
|
expectedHtml,
|
|
cases[ i ].name
|
|
);
|
|
|
|
// Now discard the replies and verify we get the original document back.
|
|
for ( j = 0; j < nodes.length; j++ ) {
|
|
modifier.removeListItem( nodes[ j ] );
|
|
}
|
|
|
|
reverseActualHtml = fixture.innerHTML;
|
|
assert.strictEqual(
|
|
reverseActualHtml,
|
|
reverseExpectedHtml,
|
|
cases[ i ].name + ' (discard replies)'
|
|
);
|
|
}
|
|
} );
|
|
|
|
QUnit.test( '#addReplyLink', function ( assert ) {
|
|
var i, j, cases, actualHtml, expectedHtml, comments, linkNode, fixture;
|
|
|
|
cases = [
|
|
{
|
|
name: 'plwiki oldparser',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/pl-big-oldparser/pl-big-oldparser.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/pl-big-oldparser/pl-big-oldparser-reply.html' ).render(),
|
|
config: require( './data/plwiki-config.json' ),
|
|
data: require( './data/plwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'enwiki oldparser',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/en-big-oldparser/en-big-oldparser.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/en-big-oldparser/en-big-oldparser-reply.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
},
|
|
{
|
|
name: 'Signatures in funny places',
|
|
dom: mw.template.get( 'test.DiscussionTools', 'cases/signatures-funny/signatures-funny.html' ).render(),
|
|
expected: mw.template.get( 'test.DiscussionTools', 'cases/signatures-funny/signatures-funny-reply.html' ).render(),
|
|
config: require( './data/enwiki-config.json' ),
|
|
data: require( './data/enwiki-data.json' )
|
|
}
|
|
];
|
|
|
|
fixture = document.getElementById( 'qunit-fixture' );
|
|
|
|
for ( i = 0; i < cases.length; i++ ) {
|
|
utils.overrideMwConfig( cases[ i ].config );
|
|
utils.overrideParserData( cases[ i ].data );
|
|
|
|
$( fixture ).empty().append( cases[ i ].expected );
|
|
expectedHtml = fixture.innerHTML;
|
|
|
|
$( fixture ).empty().append( cases[ i ].dom.clone() );
|
|
|
|
comments = parser.getComments( fixture );
|
|
parser.groupThreads( comments );
|
|
|
|
// Add a reply link to every comment.
|
|
for ( j = 0; j < comments.length; j++ ) {
|
|
if ( comments[ j ].type === 'heading' ) {
|
|
continue;
|
|
}
|
|
linkNode = document.createElement( 'a' );
|
|
linkNode.textContent = 'Reply';
|
|
linkNode.href = '#';
|
|
modifier.addReplyLink( comments[ j ], linkNode );
|
|
}
|
|
|
|
// Uncomment this to get updated content for the the "reply HTML" files, for copy/paste:
|
|
// console.log( fixture.innerHTML );
|
|
|
|
actualHtml = fixture.innerHTML.trim();
|
|
|
|
assert.strictEqual(
|
|
actualHtml,
|
|
expectedHtml,
|
|
cases[ i ].name
|
|
);
|
|
}
|
|
} );
|