mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-25 00:38:33 +00:00
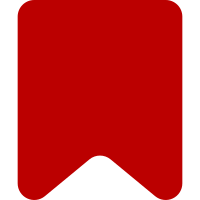
Any template that outputs wikitext spanning more than one line will break. As we can't enforce that in VE, we should just disable all templates for now. The can still be inserted in source mode, and will be eventually supported when we have multi-line syntax. Bug: T253667 Change-Id: I72a7e4c09f83bcfc2a9cc7ab33a3d5303ced851d
73 lines
1.7 KiB
JavaScript
73 lines
1.7 KiB
JavaScript
var CommentTarget = require( './CommentTarget.js' );
|
|
|
|
/**
|
|
* DiscussionTools TargetWidget class
|
|
*
|
|
* @class
|
|
* @extends ve.ui.MWTargetWidget
|
|
*
|
|
* @constructor
|
|
* @param {Object} [config] Configuration options
|
|
*/
|
|
function CommentTargetWidget( config ) {
|
|
config = $.extend( {}, {
|
|
excludeCommands: [
|
|
'heading1',
|
|
'heading2',
|
|
'heading3',
|
|
'heading4',
|
|
'heading5',
|
|
'heading6',
|
|
'insertTable',
|
|
'transclusionFromSequence' // T253667
|
|
]
|
|
}, config );
|
|
|
|
this.authors = config.authors;
|
|
|
|
// Parent constructor
|
|
CommentTargetWidget.super.call( this, config );
|
|
|
|
// Initialization
|
|
this.$element.addClass( 'dt-ui-targetWidget' );
|
|
}
|
|
|
|
/* Inheritance */
|
|
|
|
OO.inheritClass( CommentTargetWidget, ve.ui.MWTargetWidget );
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
CommentTargetWidget.prototype.createTarget = function () {
|
|
return new CommentTarget( {
|
|
// A lot of places expect ve.init.target to exist...
|
|
register: true,
|
|
toolbarGroups: this.toolbarGroups,
|
|
inTargetWidget: true,
|
|
modes: this.modes,
|
|
defaultMode: this.defaultMode
|
|
} );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
CommentTargetWidget.prototype.setDocument = function ( docOrHtml ) {
|
|
var doc = typeof docOrHtml === 'string' ? this.target.parseDocument( docOrHtml ) : docOrHtml,
|
|
// TODO: This could be upstreamed:
|
|
dmDoc = this.target.constructor.static.createModelFromDom( doc, this.target.getDefaultMode() );
|
|
|
|
// Parent method
|
|
CommentTargetWidget.super.prototype.setDocument.call( this, dmDoc );
|
|
|
|
// Remove MW specific classes as the widget is already inside the content area
|
|
this.getSurface().getView().$element.removeClass( 'mw-body-content' );
|
|
this.getSurface().$placeholder.removeClass( 'mw-body-content' );
|
|
|
|
// HACK
|
|
this.getSurface().authors = this.authors;
|
|
};
|
|
|
|
module.exports = CommentTargetWidget;
|