mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-23 18:38:18 +00:00
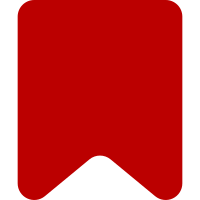
Rename ThreadItem to ContentThreadItem, then create a new ThreadItem interface containing only the methods that we'll be able to implement using only the persistently stored data (no parsing), then create a DatabaseThreadItem. Do the same for CommentItem and HeadingItem. ThreadItemSet gets a similar treatment, but it's basically only for Phan's type checking. (This is sad.) Change-Id: I1633049befe8ec169753b82eb876459af1f63fe8
39 lines
1.4 KiB
PHP
39 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools\ThreadItem;
|
|
|
|
trait ThreadItemTrait {
|
|
// phpcs:disable Squiz.WhiteSpace, MediaWiki.Commenting
|
|
// Required ThreadItem methods (listed for Phan)
|
|
abstract public function getId(): string;
|
|
abstract public function getType(): string;
|
|
abstract public function getReplies(): array;
|
|
abstract public function getLevel(): int;
|
|
// phpcs:enable
|
|
|
|
/**
|
|
* @param bool $deep Whether to include full serialized comments in the replies key
|
|
* @param callable|null $callback Function to call on the returned serialized array, which
|
|
* will be passed into the serialized replies as well if $deep is used
|
|
* @return array JSON-serializable array
|
|
*/
|
|
public function jsonSerialize( bool $deep = false, ?callable $callback = null ): array {
|
|
// The output of this method can end up in the HTTP cache (Varnish). Avoid changing it;
|
|
// and when doing so, ensure that frontend code can handle both the old and new outputs.
|
|
// See ThreadItem.static.newFromJSON in JS.
|
|
|
|
$array = [
|
|
'type' => $this->getType(),
|
|
'level' => $this->getLevel(),
|
|
'id' => $this->getId(),
|
|
'replies' => array_map( static function ( ThreadItem $comment ) use ( $deep, $callback ) {
|
|
return $deep ? $comment->jsonSerialize( $deep, $callback ) : $comment->getId();
|
|
}, $this->getReplies() )
|
|
];
|
|
if ( $callback ) {
|
|
$callback( $array, $this );
|
|
}
|
|
return $array;
|
|
}
|
|
}
|