mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-24 00:13:36 +00:00
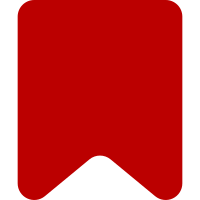
MediaWiki's PHPCS plugin requires documentation comments on all methods, unless those methods are fully typed (all parameters and return value). It turns out that almost all of our methods are fully typed already. Procedure: 1. Find: \*(\s*\*\s*(@param \??[\w\\]+(\|null)? &?\$\w+|@return \??[\w\\]+(\|null)?)\n)+\s*\*/ Replace with: */ This deletes type annotations, except those not representable as PHP type hints such as union types `a|b` or typed arrays `a[]`, or those with documentation beyond type hints, or those on functions with any other annotations. 2. Find: /\*\*/\n\s* Replace with nothing This deletes the remaining comments on methods that had no prose documentation. 3. Undo all changes that PHPCS complains about (those comments were not redundant) 4. Review the diff carefully, these regexps are imprecise :) Change-Id: Ic82e8b23f2996f44951208dbd9cfb4c8e0738dac
43 lines
1.5 KiB
PHP
43 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools\ThreadItem;
|
|
|
|
trait HeadingItemTrait {
|
|
// phpcs:disable Squiz.WhiteSpace, MediaWiki.Commenting
|
|
// Required ThreadItem methods (listed for Phan)
|
|
abstract public function getName(): string;
|
|
// Required HeadingItem methods (listed for Phan)
|
|
abstract public function getHeadingLevel(): int;
|
|
abstract public function isPlaceholderHeading(): bool;
|
|
// phpcs:enable
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @suppress PhanTraitParentReference
|
|
*/
|
|
public function jsonSerialize( bool $deep = false, ?callable $callback = null ): array {
|
|
return array_merge( [
|
|
'headingLevel' => $this->isPlaceholderHeading() ? null : $this->getHeadingLevel(),
|
|
// Used for topic subscriptions. Not added to CommentItem's yet as there is
|
|
// no use case for it.
|
|
'name' => $this->getName(),
|
|
], parent::jsonSerialize( $deep, $callback ) );
|
|
}
|
|
|
|
/**
|
|
* Check whether this heading can be used for topic subscriptions.
|
|
*/
|
|
public function isSubscribable(): bool {
|
|
return (
|
|
// Placeholder headings have nothing to attach the button to.
|
|
!$this->isPlaceholderHeading() &&
|
|
// We only allow subscribing to level 2 headings, because the user interface for sub-headings
|
|
// would be difficult to present.
|
|
$this->getHeadingLevel() === 2 &&
|
|
// Check if the name corresponds to a section that contain no comments (only sub-sections).
|
|
// They can't be distinguished from each other, so disallow subscribing.
|
|
$this->getName() !== 'h-'
|
|
);
|
|
}
|
|
}
|