mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-12 09:58:17 +00:00
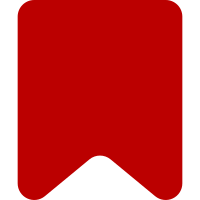
This is similar to what the JS version does. The TreeWalker and NodeFilter classes are adapted from https://github.com/Krinkle/dom-TreeWalker-polyfill (MIT license). This makes #getComments twice as fast on en-big-oldparser.html Change-Id: I2441f33e6e7bad753ac830d277e6a2e81ee8c93d
61 lines
1.5 KiB
PHP
61 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools;
|
|
|
|
use DOMNode;
|
|
use Exception;
|
|
|
|
/**
|
|
* Partial implementation of W3 DOM4 NodeFilter interface.
|
|
*
|
|
* See also:
|
|
* - https://dom.spec.whatwg.org/#interface-nodefilter
|
|
*
|
|
* Adapted from https://github.com/Krinkle/dom-TreeWalker-polyfill/blob/master/src/TreeWalker-polyfill.js
|
|
*/
|
|
class NodeFilter {
|
|
|
|
// Constants for acceptNode()
|
|
public const FILTER_ACCEPT = 1;
|
|
public const FILTER_REJECT = 2;
|
|
public const FILTER_SKIP = 3;
|
|
|
|
// Constants for whatToShow
|
|
public const SHOW_ALL = 0xFFFFFFFF;
|
|
public const SHOW_ELEMENT = 0x1;
|
|
public const SHOW_ATTRIBUTE = 0x2;
|
|
public const SHOW_TEXT = 0x4;
|
|
public const SHOW_CDATA_SECTION = 0x8;
|
|
public const SHOW_ENTITY_REFERENCE = 0x10;
|
|
public const SHOW_ENTITY = 0x20;
|
|
public const SHOW_PROCESSING_INSTRUCTION = 0x40;
|
|
public const SHOW_COMMENT = 0x80;
|
|
public const SHOW_DOCUMENT = 0x100;
|
|
public const SHOW_DOCUMENT_TYPE = 0x200;
|
|
public const SHOW_DOCUMENT_FRAGMENT = 0x400;
|
|
public const SHOW_NOTATION = 0x800;
|
|
|
|
public $filter;
|
|
|
|
private $active = false;
|
|
|
|
/**
|
|
* See https://dom.spec.whatwg.org/#dom-nodefilter-acceptnode
|
|
*
|
|
* @param DOMNode $node
|
|
* @return int Constant NodeFilter::FILTER_ACCEPT,
|
|
* NodeFilter::FILTER_REJECT or NodeFilter::FILTER_SKIP.
|
|
*/
|
|
public function acceptNode( $node ) {
|
|
if ( $this->active ) {
|
|
throw new Exception( 'DOMException: INVALID_STATE_ERR' );
|
|
}
|
|
|
|
$this->active = true;
|
|
$result = call_user_func( $this->filter, $node );
|
|
$this->active = false;
|
|
|
|
return $result;
|
|
}
|
|
}
|