mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-24 19:08:16 +00:00
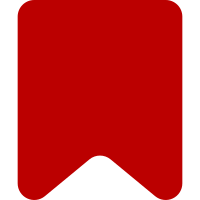
If the user talk edit or mention coincides with exactly one new comment: * Change the primary link to be a direct link to the comment * Add a text snippet to notifications that don't already include one (user talk edits that are not new sections). This is done for all such notifications, regardless of whether anyone has topic subscriptions enabled. Bug: T281590 Bug: T253082 Change-Id: I98fbca8e57845cd7c82ad533c393db953e4e5643
100 lines
2.8 KiB
PHP
100 lines
2.8 KiB
PHP
<?php
|
|
/**
|
|
* DiscussionTools echo hooks
|
|
*
|
|
* @file
|
|
* @ingroup Extensions
|
|
* @license MIT
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools\Hooks;
|
|
|
|
use EchoEvent;
|
|
use MediaWiki\Extension\DiscussionTools\Notifications\EventDispatcher;
|
|
use MediaWiki\MediaWikiServices;
|
|
use MediaWiki\Revision\RevisionRecord;
|
|
|
|
class EchoHooks {
|
|
/**
|
|
* Add notification events to Echo
|
|
*
|
|
* @param array &$notifications
|
|
* @param array &$notificationCategories
|
|
* @param array &$icons
|
|
*/
|
|
public static function onBeforeCreateEchoEvent(
|
|
array &$notifications,
|
|
array &$notificationCategories,
|
|
array &$icons
|
|
) {
|
|
$services = MediaWikiServices::getInstance();
|
|
$dtConfig = $services->getConfigFactory()->makeConfig( 'discussiontools' );
|
|
if ( !$dtConfig->get( 'DiscussionToolsEnableTopicSubscriptionBackend' ) ) {
|
|
// Topic subscriptions not available on wiki.
|
|
return;
|
|
}
|
|
|
|
$notificationCategories['dt-subscription'] = [
|
|
'priority' => 3,
|
|
'tooltip' => 'echo-pref-tooltip-dt-subscription',
|
|
];
|
|
|
|
$notifications['dt-subscribed-new-comment'] = [
|
|
'category' => 'dt-subscription',
|
|
'group' => 'interactive',
|
|
'section' => 'message',
|
|
'user-locators' => [
|
|
'MediaWiki\\Extension\\DiscussionTools\\Notifications\\EventDispatcher::locateSubscribedUsers'
|
|
],
|
|
// Exclude mentioned users and talk page owner from our notification, to avoid
|
|
// duplicate notifications for a single comment
|
|
'user-filters' => [
|
|
[
|
|
"EchoUserLocator::locateFromEventExtra",
|
|
[ "mentioned-users" ]
|
|
],
|
|
"EchoUserLocator::locateTalkPageOwner"
|
|
],
|
|
'presentation-model' =>
|
|
'MediaWiki\\Extension\\DiscussionTools\\Notifications\\SubscribedNewCommentPresentationModel',
|
|
'bundle' => [
|
|
'web' => true,
|
|
'email' => true,
|
|
'expandable' => true,
|
|
],
|
|
];
|
|
|
|
// Override default handlers
|
|
$notifications['edit-user-talk']['presentation-model'] =
|
|
'MediaWiki\\Extension\\DiscussionTools\\Notifications\\EnhancedEchoEditUserTalkPresentationModel';
|
|
$notifications['mention']['presentation-model'] =
|
|
'MediaWiki\\Extension\\DiscussionTools\\Notifications\\EnhancedEchoMentionPresentationModel';
|
|
}
|
|
|
|
/**
|
|
* @param EchoEvent $event
|
|
* @param string &$bundleString
|
|
* @return bool
|
|
*/
|
|
public static function onEchoGetBundleRules( EchoEvent $event, string &$bundleString ): bool {
|
|
switch ( $event->getType() ) {
|
|
case 'dt-subscribed-new-comment':
|
|
$bundleString = $event->getType() . '-' . $event->getExtraParam( 'subscribed-comment-name' );
|
|
break;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @param array &$events
|
|
* @param RevisionRecord $revision
|
|
* @param bool $isRevert
|
|
*/
|
|
public static function onEchoGetEventsForRevision( array &$events, RevisionRecord $revision, bool $isRevert ) {
|
|
if ( $isRevert ) {
|
|
return;
|
|
}
|
|
EventDispatcher::generateEventsForRevision( $events, $revision );
|
|
}
|
|
}
|