mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-23 10:29:11 +00:00
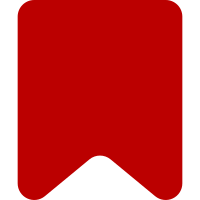
Bug: T322651 Depends-On: I86d461756398780dc24949013f35b7730a481052 Change-Id: I85ee8e6ed6eba97b94f4e4c415fbc5286c234cce
114 lines
2.9 KiB
PHP
114 lines
2.9 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools;
|
|
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItem\CommentItem;
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItem\ContentCommentItem;
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItem\ContentHeadingItem;
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItem\ContentThreadItem;
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItem\HeadingItem;
|
|
use MediaWiki\Extension\DiscussionTools\ThreadItem\ThreadItem;
|
|
use Wikimedia\Assert\Assert;
|
|
|
|
/**
|
|
* Groups thread items (headings and comments) generated by parsing a discussion page.
|
|
*/
|
|
class ContentThreadItemSet implements ThreadItemSet {
|
|
|
|
/** @var ContentThreadItem[] */
|
|
private array $threadItems = [];
|
|
/** @var ContentCommentItem[] */
|
|
private array $commentItems = [];
|
|
/** @var ContentThreadItem[][] */
|
|
private array $threadItemsByName = [];
|
|
/** @var ContentThreadItem[] */
|
|
private array $threadItemsById = [];
|
|
/** @var ContentHeadingItem[] */
|
|
private array $threads = [];
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @param ThreadItem $item
|
|
*/
|
|
public function addThreadItem( ThreadItem $item ) {
|
|
Assert::precondition( $item instanceof ContentThreadItem, 'Must be ContentThreadItem' );
|
|
|
|
$this->threadItems[] = $item;
|
|
if ( $item instanceof CommentItem ) {
|
|
$this->commentItems[] = $item;
|
|
}
|
|
if ( $item instanceof HeadingItem ) {
|
|
$this->threads[] = $item;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function isEmpty(): bool {
|
|
return !$this->threadItems;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @param ThreadItem $item
|
|
*/
|
|
public function updateIdAndNameMaps( ThreadItem $item ) {
|
|
Assert::precondition( $item instanceof ContentThreadItem, 'Must be ContentThreadItem' );
|
|
|
|
$this->threadItemsByName[ $item->getName() ][] = $item;
|
|
|
|
$this->threadItemsById[ $item->getId() ] = $item;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return ContentThreadItem[] Thread items
|
|
*/
|
|
public function getThreadItems(): array {
|
|
return $this->threadItems;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return ContentCommentItem[] Comment items
|
|
*/
|
|
public function getCommentItems(): array {
|
|
return $this->commentItems;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return ContentThreadItem[] Thread items, empty array if not found
|
|
*/
|
|
public function findCommentsByName( string $name ): array {
|
|
return $this->threadItemsByName[$name] ?? [];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return ContentThreadItem|null Thread item, null if not found
|
|
*/
|
|
public function findCommentById( string $id ): ?ThreadItem {
|
|
return $this->threadItemsById[$id] ?? null;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return ContentHeadingItem[] Tree structure of comments, top-level items are the headings.
|
|
*/
|
|
public function getThreads(): array {
|
|
return $this->threads;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @return ContentHeadingItem[] Tree structure of comments, top-level items are the headings.
|
|
*/
|
|
public function getThreadsStructured(): array {
|
|
return array_values( array_filter( $this->getThreads(), static function ( ContentThreadItem $item ) {
|
|
return $item->getParent() === null;
|
|
} ) );
|
|
}
|
|
}
|