mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-13 18:37:07 +00:00
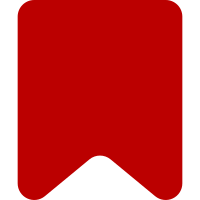
For comments posted on or after 2022-07-12 (configurable), use a shorter format, identical to the timestamps MediaWiki uses in URLs. Changing the format for already existing comments would involve difficult migrations, therefore we elect not to do it. Bug: T304595 Change-Id: I387051a6a3a1d84cfae45c3e1516db870cc8b977
84 lines
2.7 KiB
JavaScript
84 lines
2.7 KiB
JavaScript
var ThreadItem = require( './ThreadItem.js' ),
|
|
moment = require( './lib/moment-timezone/moment-timezone-with-data-1970-2030.js' );
|
|
|
|
/**
|
|
* A comment item
|
|
*
|
|
* @class CommentItem
|
|
* @extends ThreadItem
|
|
* @constructor
|
|
* @param {number} level
|
|
* @param {Object} range
|
|
* @param {Object[]} [signatureRanges] Objects describing the extent of signatures (plus
|
|
* timestamps) for this comment. There is always at least one signature, but there may be
|
|
* multiple. The author and timestamp of the comment is determined from the first signature.
|
|
* The last node in every signature range is a node containing the timestamp.
|
|
* @param {moment} [timestamp] Timestamp (Moment object)
|
|
* @param {string} [author] Comment author's username
|
|
*/
|
|
function CommentItem( level, range, signatureRanges, timestamp, author ) {
|
|
// Parent constructor
|
|
CommentItem.super.call( this, 'comment', level, range );
|
|
|
|
this.signatureRanges = signatureRanges || [];
|
|
this.timestamp = timestamp || null;
|
|
this.author = author || null;
|
|
|
|
/**
|
|
* @member {ThreadItem} Parent thread item
|
|
*/
|
|
this.parent = null;
|
|
}
|
|
|
|
OO.inheritClass( CommentItem, ThreadItem );
|
|
|
|
/**
|
|
* Get the comment timestamp in the format used in IDs and names.
|
|
*
|
|
* Depending on the date of the comment, this may use one of two formats:
|
|
*
|
|
* - For dates prior to 'DiscussionToolsTimestampFormatSwitchTime' (by default 2022-07-12):
|
|
* Uses ISO 8601 date. Almost DateTimeInterface::RFC3339_EXTENDED, but ending with 'Z' instead
|
|
* of '+00:00', like Date#toISOString in JavaScript.
|
|
*
|
|
* - For dates on or after 'DiscussionToolsTimestampFormatSwitchTime' (by default 2022-07-12):
|
|
* Uses MediaWiki timestamp (TS_MW in MediaWiki PHP code).
|
|
*
|
|
* @return {string} Comment timestamp in standard format
|
|
*/
|
|
CommentItem.prototype.getTimestampString = function () {
|
|
var dtConfig = require( './config.json' );
|
|
var switchTime = moment( dtConfig.switchTime );
|
|
if ( this.timestamp < switchTime ) {
|
|
return this.timestamp.toISOString();
|
|
} else {
|
|
return this.timestamp.format( 'YYYYMMDDHHmmss' );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* @return {HeadingItem} Closest ancestor which is a HeadingItem
|
|
*/
|
|
CommentItem.prototype.getHeading = function () {
|
|
var parent = this;
|
|
while ( parent && parent.type !== 'heading' ) {
|
|
parent = parent.parent;
|
|
}
|
|
return parent;
|
|
};
|
|
|
|
/**
|
|
* @return {HeadingItem|null} Closest heading that can be used for topic subscriptions
|
|
*/
|
|
CommentItem.prototype.getSubscribableHeading = function () {
|
|
var heading = this.getHeading();
|
|
while ( heading && heading.type === 'heading' && !heading.isSubscribable() ) {
|
|
heading = heading.parent;
|
|
}
|
|
return ( heading && heading.type === 'heading' ) ? heading : null;
|
|
};
|
|
|
|
// TODO: Implement getBodyRange/getBodyHTML/getBodyText/getMentions if required
|
|
|
|
module.exports = CommentItem;
|