mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-09-23 10:29:11 +00:00
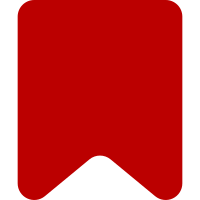
Change code to match the documented consensus formed on T321683: https://www.mediawiki.org/wiki/Manual:Coding_conventions/PHP#Exception_handling * Do not directly throw Exception, Error or MWException * Document checked exceptions with @throws * Do not document unchecked exceptions For this extension, I think it makes sense to consider DOMException an unchecked exception too (in addition to the usual LogicException and RuntimeException). Depends-On: Id07e301c3f20afa135e5469ee234a27354485652 Depends-On: I869af06896b9757af18488b916211c5a41a8c563 Depends-On: I42d9b7465d1406a22ef1b3f6d8de426c60c90e2c Change-Id: Ic9d9efd031a87fa5a93143f714f0adb20f0dd956
68 lines
1.4 KiB
PHP
68 lines
1.4 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\DiscussionTools;
|
|
|
|
use ApiBase;
|
|
use ApiMain;
|
|
use ApiUsageException;
|
|
use Wikimedia\ParamValidator\ParamValidator;
|
|
|
|
class ApiDiscussionToolsGetSubscriptions extends ApiBase {
|
|
|
|
private SubscriptionStore $subscriptionStore;
|
|
|
|
public function __construct(
|
|
ApiMain $main,
|
|
string $name,
|
|
SubscriptionStore $subscriptionStore
|
|
) {
|
|
parent::__construct( $main, $name );
|
|
$this->subscriptionStore = $subscriptionStore;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
* @throws ApiUsageException
|
|
*/
|
|
public function execute() {
|
|
$user = $this->getUser();
|
|
if ( !$user->isRegistered() ) {
|
|
$this->dieWithError( 'apierror-mustbeloggedin-generic', 'notloggedin' );
|
|
}
|
|
|
|
$params = $this->extractRequestParams();
|
|
$itemNames = $params['commentname'];
|
|
$items = $this->subscriptionStore->getSubscriptionItemsForUser(
|
|
$user,
|
|
$itemNames
|
|
);
|
|
|
|
// Ensure consistent formatting in JSON and XML formats
|
|
$this->getResult()->addIndexedTagName( 'subscriptions', 'subscription' );
|
|
$this->getResult()->addArrayType( 'subscriptions', 'kvp', 'name' );
|
|
|
|
foreach ( $items as $item ) {
|
|
$this->getResult()->addValue( 'subscriptions', $item->getItemName(), $item->getState() );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getAllowedParams() {
|
|
return [
|
|
'commentname' => [
|
|
ParamValidator::PARAM_REQUIRED => true,
|
|
ParamValidator::PARAM_ISMULTI => true,
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function isInternal() {
|
|
return true;
|
|
}
|
|
}
|