mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/DiscussionTools
synced 2024-11-11 17:02:28 +00:00
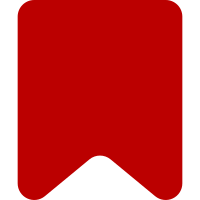
I think the issues in T329299 are caused by partially autosaved comments. We store data in multiple localStorage keys, and if some of them are stored but others are not (due to exceeding storage quota), our code can't handle the inconsistent state. We already have a wrapper around localStorage that tries to cover up these issues. Change it so that all values specific to an instance of a reply tool are stored under one localStorage key. This ensures that all updates consistently succeed or fail, with no partially stored state. One of the reasons we haven't done this is because this requires the whole data to be serialized to JSON every time, but our experience with VE change 4355d697aa shows that this is fast enough. Extra changes: * Remove storagePrefix, now redundant * Remove use of createConflictableStorage, now redundant * Prefix the key with 'mw' as advised by mw.storage documentation * Use ES6 syntax for the new code (just for fun) * Use consistent expiry (T339042) Bug: T329299 Change-Id: I347115f7187fd7d6afd9c6f368441e262154233b
76 lines
2 KiB
JavaScript
76 lines
2 KiB
JavaScript
/**
|
|
* MemoryStorage is a wrapper around mw.SafeStorage objects.
|
|
*
|
|
* It provides two mechanisms to improve their behavior when the underlying storage mechanism fails
|
|
* (e.g. quota exceeded):
|
|
*
|
|
* - Duplicating their contents in memory, so that the storage can be relied on before the page has
|
|
* been reloaded.
|
|
* - Storing all keys in a single underlying key, to make the stores are atomic: either all values
|
|
* are stored or none.
|
|
*
|
|
* This has two additional consequences which are convenient for our use case:
|
|
*
|
|
* - All keys share the same expiry time, removing the need to specify it repeatedly.
|
|
* - When multiple processes try to write the same keys, all keys are overwritten, so that we don't
|
|
* have to worry about inconsistent data.
|
|
*
|
|
* @example
|
|
* var sessionStorage = new MemoryStorage( mw.storage.session, 'myprefix' );
|
|
* var localStorage = new MemoryStorage( mw.storage, 'myprefix' );
|
|
*/
|
|
class MemoryStorage {
|
|
/**
|
|
* @param {mw.SafeStorage} backend
|
|
* @param {string} key Key name to store under
|
|
* @param {number} [expiry] Number of seconds after which this item can be deleted
|
|
*/
|
|
constructor( backend, key, expiry ) {
|
|
this.backend = backend;
|
|
this.key = key;
|
|
this.expiry = expiry;
|
|
|
|
this.data = this.backend.getObject( this.key ) || {};
|
|
}
|
|
|
|
get( key ) {
|
|
if ( Object.prototype.hasOwnProperty.call( this.data, key ) ) {
|
|
return this.data[ key ];
|
|
}
|
|
return null;
|
|
}
|
|
|
|
set( key, value ) {
|
|
this.data[ key ] = value;
|
|
this.backend.setObject( this.key, this.data, this.expiry );
|
|
return true;
|
|
}
|
|
|
|
remove( key ) {
|
|
delete this.data[ key ];
|
|
if ( Object.keys( this.data ).length === 0 ) {
|
|
this.backend.remove( this.key );
|
|
} else {
|
|
this.backend.setObject( this.key, this.data, this.expiry );
|
|
}
|
|
return true;
|
|
}
|
|
|
|
clear() {
|
|
this.data = {};
|
|
this.backend.remove( this.key );
|
|
}
|
|
|
|
// For compatibility with mw.SafeStorage API
|
|
getObject( key ) {
|
|
return this.get( key );
|
|
}
|
|
|
|
// For compatibility with mw.SafeStorage API
|
|
setObject( key, value ) {
|
|
return this.set( key, value );
|
|
}
|
|
}
|
|
|
|
module.exports = MemoryStorage;
|