mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CookieWarning
synced 2024-11-27 15:40:16 +00:00
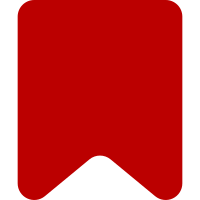
This cleans up the hook file a lot and movesthe logic to seperate services provided by MediaWikiServices. This also removes some setters and passed- around variables and stuff. Also fixes the unit tests by not querying external services anymore. Change-Id: I0c3c0b7f2f5bd68aaad624e8e2dcad9bdcf97770
66 lines
1.8 KiB
PHP
66 lines
1.8 KiB
PHP
<?php
|
|
/**
|
|
* GeoLocation implementation
|
|
*/
|
|
|
|
/**
|
|
* Implements the GeoLocation class, which allows to locate the user based on the IP address.
|
|
*/
|
|
class GeoLocation {
|
|
private $config;
|
|
private $countryCode;
|
|
|
|
/**
|
|
* @param Config $config
|
|
*/
|
|
public function __construct( Config $config ) {
|
|
$this->config = $config;
|
|
}
|
|
|
|
/**
|
|
* Returns the country code, if the last call to self::locate() returned true. Otherwise, NULL.
|
|
*
|
|
* @return null|string
|
|
*/
|
|
public function getCountryCode() {
|
|
return $this->countryCode;
|
|
}
|
|
|
|
/**
|
|
* Tries to locate the IP address set with self::setIP() using the geolocation service
|
|
* configured with the $wgCookieWarningGeoIPServiceURL configuration variable. If the config
|
|
* isn't set, this function returns NULL. If the config is set, but the URL is invalid or an
|
|
* other problem occures which resulted in a failed locating process, this function returns
|
|
* false, otherwise it returns true.
|
|
*
|
|
* @param string $ip The IP address to lookup
|
|
* @return bool|null NULL if no geolocation service configured, false on error, true otherwise.
|
|
* @throws ConfigException
|
|
*/
|
|
public function locate( $ip ) {
|
|
$this->countryCode = null;
|
|
if ( !IP::isValid( $ip ) ) {
|
|
throw new InvalidArgumentException( "$ip is not a valid IP address." );
|
|
}
|
|
if ( !$this->config->get( 'CookieWarningGeoIPServiceURL' ) ) {
|
|
return null;
|
|
}
|
|
$requestUrl = $this->config->get( 'CookieWarningGeoIPServiceURL' );
|
|
if ( substr( $requestUrl, -1 ) !== '/' ) {
|
|
$requestUrl .= '/';
|
|
}
|
|
$json = Http::get( $requestUrl . $ip, [
|
|
'timeout' => '2'
|
|
] );
|
|
if ( !$json ) {
|
|
return false;
|
|
}
|
|
$returnObject = json_decode( $json );
|
|
if ( $returnObject === null || !property_exists( $returnObject, 'country_code' ) ) {
|
|
return false;
|
|
}
|
|
$this->countryCode = $returnObject->country_code;
|
|
return true;
|
|
}
|
|
}
|