mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CookieWarning
synced 2024-11-23 22:03:41 +00:00
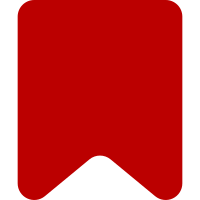
This should make the code a bit more structured and easier to navigate through. It also removes some lines from extension.json as the autoload classes list is replaced by an autoload namespaces property. This also changes the minimum requirement of MediaWiki to 1.31 for this extension to work. Change-Id: I249ccc3035297c99202a5f83fcc8d3fda68b682c
75 lines
1.9 KiB
PHP
75 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace CookieWarning;
|
|
|
|
/**
|
|
* GeoLocation implementation
|
|
*/
|
|
|
|
use Config;
|
|
use ConfigException;
|
|
use Http;
|
|
use InvalidArgumentException;
|
|
use IP;
|
|
|
|
/**
|
|
* Implements the GeoLocation class, which allows to locate the user based on the IP address.
|
|
*/
|
|
class GeoLocation {
|
|
private $config;
|
|
private $countryCode;
|
|
|
|
/**
|
|
* @param Config $config
|
|
*/
|
|
public function __construct( Config $config ) {
|
|
$this->config = $config;
|
|
}
|
|
|
|
/**
|
|
* Returns the country code, if the last call to self::locate() returned true. Otherwise, NULL.
|
|
*
|
|
* @return null|string
|
|
*/
|
|
public function getCountryCode() {
|
|
return $this->countryCode;
|
|
}
|
|
|
|
/**
|
|
* Tries to locate the IP address set with self::setIP() using the geolocation service
|
|
* configured with the $wgCookieWarningGeoIPServiceURL configuration variable. If the config
|
|
* isn't set, this function returns NULL. If the config is set, but the URL is invalid or an
|
|
* other problem occures which resulted in a failed locating process, this function returns
|
|
* false, otherwise it returns true.
|
|
*
|
|
* @param string $ip The IP address to lookup
|
|
* @return bool|null NULL if no geolocation service configured, false on error, true otherwise.
|
|
* @throws ConfigException
|
|
*/
|
|
public function locate( $ip ) {
|
|
$this->countryCode = null;
|
|
if ( !IP::isValid( $ip ) ) {
|
|
throw new InvalidArgumentException( "$ip is not a valid IP address." );
|
|
}
|
|
if ( !$this->config->get( 'CookieWarningGeoIPServiceURL' ) ) {
|
|
return null;
|
|
}
|
|
$requestUrl = $this->config->get( 'CookieWarningGeoIPServiceURL' );
|
|
if ( substr( $requestUrl, -1 ) !== '/' ) {
|
|
$requestUrl .= '/';
|
|
}
|
|
$json = Http::get( $requestUrl . $ip, [
|
|
'timeout' => '2'
|
|
] );
|
|
if ( !$json ) {
|
|
return false;
|
|
}
|
|
$returnObject = json_decode( $json );
|
|
if ( $returnObject === null || !property_exists( $returnObject, 'country_code' ) ) {
|
|
return false;
|
|
}
|
|
$this->countryCode = $returnObject->country_code;
|
|
return true;
|
|
}
|
|
}
|