mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/ConfirmEdit
synced 2024-11-12 01:11:15 +00:00
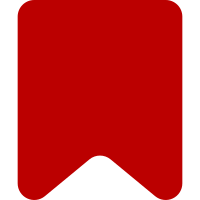
Also remove references to "two words" from ReCaptcha labels. The captcha image doesn't always contain two words. Bug: T110302 Change-Id: I544656289480056152a1db195babb6dadf29bc71
75 lines
2.5 KiB
PHP
75 lines
2.5 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Creates a ReCaptcha v2 widget. Does not return any data; handling the data submitted by the
|
|
* widget is callers' responsibility.
|
|
*/
|
|
class HTMLReCaptchaNoCaptchaField extends HTMLFormField {
|
|
/** @var string Public key parameter to be passed to ReCaptcha. */
|
|
protected $key;
|
|
|
|
/** @var string Error returned by ReCaptcha in the previous round. */
|
|
protected $error;
|
|
|
|
/**
|
|
* Parameters:
|
|
* - key: (string, required) ReCaptcha public key
|
|
* - error: (string) ReCaptcha error from previous round
|
|
* @param array $params
|
|
*/
|
|
public function __construct( array $params ) {
|
|
$params += [ 'error' => null ];
|
|
parent::__construct( $params );
|
|
|
|
$this->key = $params['key'];
|
|
$this->error = $params['error'];
|
|
|
|
$this->mName = 'g-recaptcha-response';
|
|
}
|
|
|
|
public function getInputHTML( $value ) {
|
|
$out = $this->mParent->getOutput();
|
|
$lang = htmlspecialchars( urlencode( $this->mParent->getLanguage()->getCode() ) );
|
|
|
|
// Insert reCAPTCHA script, in display language, if available.
|
|
// Language falls back to the browser's display language.
|
|
// See https://developers.google.com/recaptcha/docs/faq
|
|
$out->addHeadItem(
|
|
'g-recaptchascript',
|
|
"<script src=\"https://www.google.com/recaptcha/api.js?hl={$lang}\" async defer></script>"
|
|
);
|
|
$output = Html::element( 'div', [
|
|
'class' => [
|
|
'g-recaptcha',
|
|
'mw-confirmedit-captcha-fail' => !!$this->error,
|
|
],
|
|
'data-sitekey' => $this->key,
|
|
] );
|
|
$htmlUrlencoded = htmlspecialchars( urlencode( $this->key ) );
|
|
$output .= <<<HTML
|
|
<noscript>
|
|
<div>
|
|
<div style="width: 302px; height: 422px; position: relative;">
|
|
<div style="width: 302px; height: 422px; position: absolute;">
|
|
<iframe src="https://www.google.com/recaptcha/api/fallback?k={$htmlUrlencoded}&hl={$lang}"
|
|
frameborder="0" scrolling="no"
|
|
style="width: 302px; height:422px; border-style: none;">
|
|
</iframe>
|
|
</div>
|
|
</div>
|
|
<div style="width: 300px; height: 60px; border-style: none;
|
|
bottom: 12px; left: 25px; margin: 0px; padding: 0px; right: 25px;
|
|
background: #f9f9f9; border: 1px solid #c1c1c1; border-radius: 3px;">
|
|
<textarea id="g-recaptcha-response" name="g-recaptcha-response"
|
|
class="g-recaptcha-response"
|
|
style="width: 250px; height: 40px; border: 1px solid #c1c1c1;
|
|
margin: 10px 25px; padding: 0px; resize: none;" >
|
|
</textarea>
|
|
</div>
|
|
</div>
|
|
</noscript>
|
|
HTML;
|
|
return $output;
|
|
}
|
|
}
|