mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/ConfirmEdit
synced 2024-12-18 19:02:00 +00:00
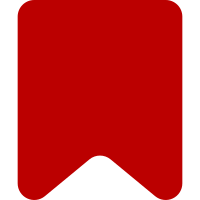
In this patch, we can now make use of MicroStash only and drop dead code. At this point, we're sure that there are no captchas in the main stash, freeing up this memory for other requests to use. Bug: T336004 Change-Id: I6aa69636f2f94e3bd18afc66eac37146d00771d1
59 lines
1.2 KiB
PHP
59 lines
1.2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\ConfirmEdit\Store;
|
|
|
|
use BagOStuff;
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
class CaptchaCacheStore extends CaptchaStore {
|
|
/** @var BagOStuff */
|
|
private $store;
|
|
|
|
public function __construct() {
|
|
parent::__construct();
|
|
|
|
$services = MediaWikiServices::getInstance();
|
|
$this->store = $services->getMicroStash();
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function store( $index, $info ) {
|
|
global $wgCaptchaSessionExpiration;
|
|
|
|
$store = $this->store;
|
|
$store->set(
|
|
$store->makeKey( 'captcha', $index ),
|
|
$info,
|
|
$wgCaptchaSessionExpiration,
|
|
// Assume the write will reach the master DC before the user sends the
|
|
// HTTP POST request attempted to solve the captcha and perform an action
|
|
$store::WRITE_BACKGROUND
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function retrieve( $index ) {
|
|
$store = $this->store;
|
|
|
|
return $store->get( $store->makeKey( 'captcha', $index ) );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function clear( $index ) {
|
|
$store = $this->store;
|
|
$store->delete( $store->makeKey( 'captcha', $index ) );
|
|
}
|
|
|
|
public function cookiesNeeded() {
|
|
return false;
|
|
}
|
|
}
|
|
|
|
class_alias( CaptchaCacheStore::class, 'CaptchaCacheStore' );
|