mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/ConfirmEdit
synced 2024-11-15 11:59:33 +00:00
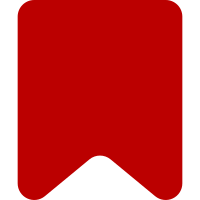
The ReCaptcha module was not injecting its parameters at all, so they were not showing up in the auto-generated help. This is now fixed. Also, the API recently added a new parameter to the APIGetAllowedParams hook to differentiate between fetching the allowed parameter list for help output and fetching it for processing within the module. ConfirmEdit only needs to inject the parameters for the former, so it should check this parameter if available. Change-Id: Ia8c9a8b882ee3480b71bfb3f2345475506549819
89 lines
2.3 KiB
PHP
89 lines
2.3 KiB
PHP
<?php
|
|
|
|
class ConfirmEditHooks {
|
|
/**
|
|
* Get the global Captcha instance
|
|
*
|
|
* @return Captcha|SimpleCaptcha
|
|
*/
|
|
static function getInstance() {
|
|
global $wgCaptcha, $wgCaptchaClass;
|
|
|
|
static $done = false;
|
|
|
|
if ( !$done ) {
|
|
$done = true;
|
|
$wgCaptcha = new $wgCaptchaClass;
|
|
}
|
|
|
|
return $wgCaptcha;
|
|
}
|
|
|
|
static function confirmEditMerged( $editPage, $newtext ) {
|
|
return self::getInstance()->confirmEditMerged( $editPage, $newtext );
|
|
}
|
|
|
|
static function confirmEditAPI( $editPage, $newtext, &$resultArr ) {
|
|
return self::getInstance()->confirmEditAPI( $editPage, $newtext, $resultArr );
|
|
}
|
|
|
|
static function injectUserCreate( &$template ) {
|
|
return self::getInstance()->injectUserCreate( $template );
|
|
}
|
|
|
|
static function confirmUserCreate( $u, &$message ) {
|
|
return self::getInstance()->confirmUserCreate( $u, $message );
|
|
}
|
|
|
|
static function triggerUserLogin( $user, $password, $retval ) {
|
|
return self::getInstance()->triggerUserLogin( $user, $password, $retval );
|
|
}
|
|
|
|
static function injectUserLogin( &$template ) {
|
|
return self::getInstance()->injectUserLogin( $template );
|
|
}
|
|
|
|
static function confirmUserLogin( $u, $pass, &$retval ) {
|
|
return self::getInstance()->confirmUserLogin( $u, $pass, $retval );
|
|
}
|
|
|
|
static function injectEmailUser( &$form ) {
|
|
return self::getInstance()->injectEmailUser( $form );
|
|
}
|
|
|
|
static function confirmEmailUser( $from, $to, $subject, $text, &$error ) {
|
|
return self::getInstance()->confirmEmailUser( $from, $to, $subject, $text, $error );
|
|
}
|
|
|
|
// Default $flags to 1 for backwards-compatible behavior
|
|
public static function APIGetAllowedParams( &$module, &$params, $flags = 1 ) {
|
|
return self::getInstance()->APIGetAllowedParams( $module, $params, $flags );
|
|
}
|
|
|
|
public static function APIGetParamDescription( &$module, &$desc ) {
|
|
return self::getInstance()->APIGetParamDescription( $module, $desc );
|
|
}
|
|
}
|
|
|
|
class CaptchaSpecialPage extends UnlistedSpecialPage {
|
|
public function __construct() {
|
|
parent::__construct( 'Captcha' );
|
|
}
|
|
|
|
function execute( $par ) {
|
|
$this->setHeaders();
|
|
|
|
$instance = ConfirmEditHooks::getInstance();
|
|
|
|
switch( $par ) {
|
|
case "image":
|
|
if ( method_exists( $instance, 'showImage' ) ) {
|
|
return $instance->showImage();
|
|
}
|
|
case "help":
|
|
default:
|
|
return $instance->showHelp();
|
|
}
|
|
}
|
|
}
|