mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/ConfirmEdit
synced 2024-11-15 11:59:33 +00:00
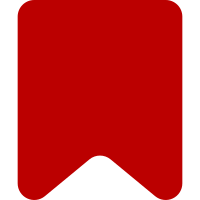
The jQuery selector to find the form only worked for create account; it now works for the captchas on login password failures and on page editing. Remove two debugging console.log() lines and fix some JSHint errors. Rename the message for login captchas from the unused asirra-badpass to the correct asirra-badlogin. Bug: 39536 Change-Id: I92c3cb93337d4a704221d5a0e50ed56ae3b6b1cf
55 lines
1.7 KiB
JavaScript
55 lines
1.7 KiB
JavaScript
/*======================================================================*\
|
|
|| #################################################################### ||
|
|
|| # Asirra module for ConfirmEdit by Bachsau # ||
|
|
|| # ---------------------------------------------------------------- # ||
|
|
|| # This code is released into public domain, in the hope that it # ||
|
|
|| # will be useful, but without any warranty. # ||
|
|
|| # ------------ YOU CAN DO WITH IT WHATEVER YOU LIKE! ------------- # ||
|
|
|| #################################################################### ||
|
|
\*======================================================================*/
|
|
|
|
jQuery( function( $ ) {
|
|
// Selectors for create account, login, and page edit forms.
|
|
var asirraform = $( 'form#userlogin2, #userloginForm form, form#editform' );
|
|
var submitButtonClicked = document.createElement("input");
|
|
var passThroughFormSubmit = false;
|
|
|
|
function PrepareSubmit() {
|
|
submitButtonClicked.type = "hidden";
|
|
var inputFields = asirraform.find( "input" );
|
|
for (var i=0; i<inputFields.length; i++) {
|
|
if (inputFields[i].type === "submit") {
|
|
inputFields[i].onclick = function(event) {
|
|
submitButtonClicked.name = this.name;
|
|
submitButtonClicked.value = this.value;
|
|
}
|
|
}
|
|
}
|
|
|
|
asirraform.submit( function() {
|
|
return MySubmitForm();
|
|
} );
|
|
}
|
|
|
|
function MySubmitForm() {
|
|
if (passThroughFormSubmit) {
|
|
return true;
|
|
}
|
|
Asirra_CheckIfHuman(HumanCheckComplete);
|
|
return false;
|
|
}
|
|
|
|
function HumanCheckComplete(isHuman) {
|
|
if (!isHuman) {
|
|
window.alert( mediaWiki.msg( 'asirra-failed' ) );
|
|
} else {
|
|
asirraform.append(submitButtonClicked);
|
|
passThroughFormSubmit = true;
|
|
asirraform.submit();
|
|
}
|
|
}
|
|
|
|
PrepareSubmit();
|
|
|
|
} );
|