mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/ConfirmEdit
synced 2024-11-15 11:59:33 +00:00
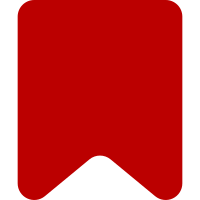
Also update MathCaptcha so that it works with recent versions of Math (and breaks with old ones). Also fix MathCaptcha API output, which used to send the question in plaintext. Bug: T110302 Change-Id: I0da671a546700110d789b79a3089460abd9cce3b Depends-On: I8b52ec8ddf494f23941807638f149f15b5e46b0c
117 lines
3.2 KiB
PHP
117 lines
3.2 KiB
PHP
<?php
|
|
|
|
class ReCaptcha extends SimpleCaptcha {
|
|
// used for recaptcha-edit, recaptcha-addurl, recaptcha-badlogin, recaptcha-createaccount,
|
|
// recaptcha-create, recaptcha-sendemail via getMessage()
|
|
protected static $messagePrefix = 'recaptcha-';
|
|
|
|
// reCAPTHCA error code returned from recaptcha_check_answer
|
|
private $recaptcha_error = null;
|
|
|
|
/**
|
|
* Displays the reCAPTCHA widget.
|
|
* If $this->recaptcha_error is set, it will display an error in the widget.
|
|
* @param OutputPage $out
|
|
*/
|
|
function getForm( OutputPage $out, $tabIndex = 1 ) {
|
|
global $wgReCaptchaPublicKey, $wgReCaptchaTheme;
|
|
|
|
$useHttps = ( isset( $_SERVER['HTTPS'] ) && $_SERVER['HTTPS'] == 'on' );
|
|
$js = 'var RecaptchaOptions = ' . Xml::encodeJsVar(
|
|
[ 'theme' => $wgReCaptchaTheme, 'tabindex' => $tabIndex ]
|
|
);
|
|
|
|
return Html::inlineScript(
|
|
$js
|
|
) . recaptcha_get_html( $wgReCaptchaPublicKey, $this->recaptcha_error, $useHttps );
|
|
}
|
|
|
|
/**
|
|
* Calls the library function recaptcha_check_answer to verify the users input.
|
|
* Sets $this->recaptcha_error if the user is incorrect.
|
|
* @return boolean
|
|
*
|
|
*/
|
|
function passCaptcha( $_, $__ ) {
|
|
global $wgReCaptchaPrivateKey, $wgRequest;
|
|
|
|
// API is hardwired to return wpCaptchaId and wpCaptchaWord,
|
|
// so use that if the standard two are empty
|
|
$challenge = $wgRequest->getVal(
|
|
'recaptcha_challenge_field', $wgRequest->getVal( 'wpCaptchaId' )
|
|
);
|
|
$response = $wgRequest->getVal(
|
|
'recaptcha_response_field', $wgRequest->getVal( 'wpCaptchaWord' )
|
|
);
|
|
|
|
if ( $response === null ) {
|
|
// new captcha session
|
|
return false;
|
|
}
|
|
|
|
$ip = $wgRequest->getIP();
|
|
|
|
$recaptcha_response = recaptcha_check_answer(
|
|
$wgReCaptchaPrivateKey,
|
|
$ip,
|
|
$challenge,
|
|
$response
|
|
);
|
|
|
|
if ( !$recaptcha_response->is_valid ) {
|
|
$this->recaptcha_error = $recaptcha_response->error;
|
|
return false;
|
|
}
|
|
|
|
$recaptcha_error = null;
|
|
|
|
return true;
|
|
|
|
}
|
|
|
|
function addCaptchaAPI( &$resultArr ) {
|
|
$resultArr['captcha'] = $this->describeCaptchaType();
|
|
$resultArr['captcha']['error'] = $this->recaptcha_error;
|
|
}
|
|
|
|
public function describeCaptchaType() {
|
|
global $wgReCaptchaPublicKey;
|
|
return [
|
|
'type' => 'recaptcha',
|
|
'mime' => 'image/png',
|
|
'key' => $wgReCaptchaPublicKey,
|
|
];
|
|
}
|
|
|
|
public function APIGetAllowedParams( &$module, &$params, $flags ) {
|
|
if ( $flags && $this->isAPICaptchaModule( $module ) ) {
|
|
if ( defined( 'ApiBase::PARAM_HELP_MSG' ) ) {
|
|
$params['recaptcha_challenge_field'] = [
|
|
ApiBase::PARAM_HELP_MSG => 'recaptcha-apihelp-param-recaptcha_challenge_field',
|
|
];
|
|
$params['recaptcha_response_field'] = [
|
|
ApiBase::PARAM_HELP_MSG => 'recaptcha-apihelp-param-recaptcha_response_field',
|
|
];
|
|
} else {
|
|
// @todo: Remove this branch when support for MediaWiki < 1.25 is dropped
|
|
$params['recaptcha_challenge_field'] = null;
|
|
$params['recaptcha_response_field'] = null;
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @deprecated since MediaWiki 1.25
|
|
*/
|
|
public function APIGetParamDescription( &$module, &$desc ) {
|
|
if ( $this->isAPICaptchaModule( $module ) ) {
|
|
$desc['recaptcha_challenge_field'] = 'Field from the ReCaptcha widget';
|
|
$desc['recaptcha_response_field'] = 'Field from the ReCaptcha widget';
|
|
}
|
|
|
|
return true;
|
|
}
|
|
}
|