mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CodeMirror
synced 2024-12-18 16:50:36 +00:00
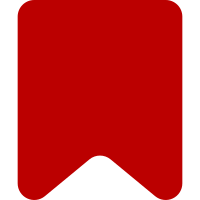
Popular extensions like Charinsert use this method to wrap text around a selection. This patch adds support for multiple selections in CM6. Some options to encapsulateSelection do not yet have explicit support here, such as 'peri', but it's unclear if they are truly needed. Bug: T211205 Change-Id: Idc0abb64eb036fa4a60382aca401d1dba1722405
111 lines
3.7 KiB
JavaScript
111 lines
3.7 KiB
JavaScript
'use strict';
|
|
|
|
const assert = require( 'assert' ),
|
|
EditPage = require( '../pageobjects/edit.page' ),
|
|
FixtureContent = require( '../fixturecontent' ),
|
|
LoginPage = require( 'wdio-mediawiki/LoginPage' ),
|
|
UserPreferences = require( '../userpreferences' ),
|
|
Util = require( 'wdio-mediawiki/Util' );
|
|
|
|
describe( 'CodeMirror textSelection for the wikitext 2010 editor', () => {
|
|
let title;
|
|
|
|
before( async () => {
|
|
title = Util.getTestString( 'CodeMirror-fixture1-' );
|
|
await LoginPage.loginAdmin();
|
|
await FixtureContent.createFixturePage( title );
|
|
await UserPreferences.enableWikitext2010EditorWithCodeMirror();
|
|
await EditPage.openForEditing( title, true );
|
|
await EditPage.wikiEditorToolbar.waitForDisplayed();
|
|
await EditPage.clickText();
|
|
} );
|
|
|
|
// Content is "[]{{template}}"
|
|
it( 'sets and gets the correct text when using setContents and getContents', async () => {
|
|
await browser.execute( () => $( '.cm-editor' ).textSelection( 'setContents', 'foobar' ) );
|
|
assert.strictEqual(
|
|
await browser.execute( () => $( '.cm-editor' ).textSelection( 'getContents' ) ),
|
|
'foobar'
|
|
);
|
|
} );
|
|
|
|
// Content is now "foobar"
|
|
it( 'sets and gets the correct selection when using setSelection and getSelection', async () => {
|
|
await browser.execute( () => {
|
|
$( '.cm-editor' ).textSelection( 'setSelection', { start: 3, end: 6 } );
|
|
} );
|
|
assert.strictEqual(
|
|
await browser.execute( () => {
|
|
return $( '.cm-editor' ).textSelection( 'getSelection' );
|
|
} ),
|
|
'bar'
|
|
);
|
|
} );
|
|
|
|
it( 'correctly replaces the selected text when using replaceSelection', async () => {
|
|
await browser.execute( () => $( '.cm-editor' ).textSelection( 'replaceSelection', 'baz' ) );
|
|
assert.strictEqual(
|
|
await browser.execute( () => $( '.cm-editor' ).textSelection( 'getContents' ) ),
|
|
'foobaz'
|
|
);
|
|
} );
|
|
|
|
// Content is now "foobaz"
|
|
it( 'returns the correct values for getCaretPosition', async () => {
|
|
await browser.execute( () => {
|
|
$( '.cm-editor' ).textSelection( 'setSelection', { start: 3, end: 6 } );
|
|
} );
|
|
assert.strictEqual(
|
|
await browser.execute( () => $( '.cm-editor' ).textSelection( 'getCaretPosition' ) ),
|
|
6
|
|
);
|
|
assert.deepStrictEqual(
|
|
await browser.execute( () => {
|
|
return $( '.cm-editor' ).textSelection( 'getCaretPosition', { startAndEnd: true } );
|
|
} ),
|
|
[ 3, 6 ]
|
|
);
|
|
} );
|
|
|
|
it( 'correctly wraps the selected text when using encapsulateSelection', async function () {
|
|
await browser.execute( () => {
|
|
$( '.cm-editor' ).textSelection( 'setContents', 'foobaz' )
|
|
.textSelection( 'encapsulateSelection', {
|
|
selectionStart: 0,
|
|
selectionEnd: 6,
|
|
pre: '<div>',
|
|
post: '</div>'
|
|
} );
|
|
} );
|
|
assert.strictEqual(
|
|
await browser.execute( () => $( '.cm-editor' ).textSelection( 'getContents' ) ),
|
|
'<div>foobaz</div>'
|
|
);
|
|
} );
|
|
|
|
// Content is now "<div>foobaz</div>"
|
|
it( 'scrolls to the correct place when using scrollToCaretPosition', async () => {
|
|
await browser.execute( () => {
|
|
const $cmEditor = $( '.cm-editor' );
|
|
$cmEditor.textSelection( 'setContents', 'foobar\n'.repeat( 50 ) );
|
|
// Ensure caret is at the top.
|
|
$cmEditor.textSelection( 'setSelection', { start: 0 } );
|
|
// Force scrolling to the bottom.
|
|
$( '.cm-scroller' )[ 0 ].scrollTop = 5000;
|
|
// Use textSelection to scroll back to caret.
|
|
$cmEditor.textSelection( 'scrollToCaretPosition' );
|
|
} );
|
|
assert.strictEqual(
|
|
await browser.execute( () => $( '.cm-scroller' ).scrollTop() ),
|
|
0
|
|
);
|
|
} );
|
|
|
|
// Content is now "foobar\n" repeated 50 times.
|
|
it( 'retains the contents after turning CodeMirror off', async () => {
|
|
await EditPage.legacyCodeMirrorButton.click();
|
|
await EditPage.legacyTextInput.waitForDisplayed();
|
|
assert.match( await EditPage.legacyTextInput.getValue(), /foobar/ );
|
|
} );
|
|
} );
|