mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CodeMirror
synced 2024-12-02 17:56:15 +00:00
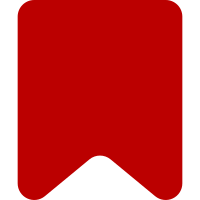
Includes a bit untangling of the setup. Concurrent tests should not overwrite each others user settings so one of the tests gets it's own user. Bug: T337862 Change-Id: Iae245063932d4c5d9e6b61c1fe102505d70c1039
50 lines
1.5 KiB
JavaScript
50 lines
1.5 KiB
JavaScript
'use strict';
|
|
|
|
const Page = require( 'wdio-mediawiki/Page' );
|
|
|
|
// Copied from mediawiki-core edit.page.js
|
|
class EditPage extends Page {
|
|
async openForEditing( title ) {
|
|
await super.openTitle( title, { action: 'edit', vehidebetadialog: 1, hidewelcomedialog: 1 } );
|
|
}
|
|
|
|
get wikiEditorToolbar() { return $( '#wikiEditor-ui-toolbar' ); }
|
|
get legacyTextInput() { return $( '#wpTextbox1' ); }
|
|
async clickText() {
|
|
if ( await this.visualEditorSave.isDisplayed() ) {
|
|
await this.visualEditorSurface.click();
|
|
} else if ( await this.legacyTextInput.isDisplayed() ) {
|
|
await this.legacyTextInput.click();
|
|
} else {
|
|
// Click the container, if using WikiEditor etc.
|
|
await this.legacyTextInput.parentElement().click();
|
|
}
|
|
}
|
|
|
|
get visualEditorSave() { return $( '.ve-ui-toolbar-saveButton' ); }
|
|
get visualEditorSurface() { return $( '.ve-ui-surface-source' ); }
|
|
|
|
async cursorToPosition( index ) {
|
|
await this.clickText();
|
|
|
|
// Second "Control" deactivates the modifier.
|
|
const keys = [ 'Control', 'Home', 'Control' ];
|
|
for ( let i = 0; i < index; i++ ) {
|
|
keys.push( 'ArrowRight' );
|
|
}
|
|
await browser.keys( keys );
|
|
}
|
|
|
|
get highlightedBrackets() { return $$( '.CodeMirror-line .cm-mw-matchingbracket' ); }
|
|
|
|
async getHighlightedMatchingBrackets() {
|
|
await this.highlightedBrackets[ 0 ].waitForDisplayed();
|
|
const matchingTexts = await this.highlightedBrackets.map( function ( el ) {
|
|
return el.getText();
|
|
} );
|
|
return matchingTexts.join( '' );
|
|
}
|
|
}
|
|
|
|
module.exports = new EditPage();
|