mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-11-15 02:55:04 +00:00
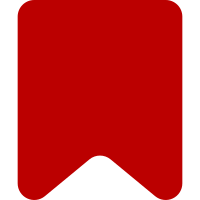
This turns two methods with side-effects into more pure functions with more obvious input and output. buildSearchIndex rebuilds the internal search index from the internalList. buildSearchResults filters and creates the result widgets the user will see. This patch really only moves existing code around but doesn't change anything. Except that this.built is set before onQueryChange is called, not after. This avoids potential endless loops in case onQueryChange happens to trigger buildIndex again. Bug: T356871 Change-Id: Ib80a2dcb85779d64bec53caf90c49879d0ea2258
93 lines
3.1 KiB
JavaScript
93 lines
3.1 KiB
JavaScript
'use strict';
|
|
|
|
QUnit.module( 've.ui.MWReferenceSearchWidget (Cite)', ve.test.utils.newMwEnvironment() );
|
|
|
|
QUnit.test( 'buildIndex', function ( assert ) {
|
|
const widget = new ve.ui.MWReferenceSearchWidget();
|
|
widget.internalList = { getNodeGroups: () => ( {} ) };
|
|
assert.false( widget.built );
|
|
|
|
widget.buildIndex();
|
|
assert.true( widget.built );
|
|
assert.deepEqual( widget.index, [] );
|
|
|
|
widget.onInternalListUpdate( [ 'mwReference/' ] );
|
|
assert.false( widget.built );
|
|
assert.deepEqual( widget.index, [] );
|
|
|
|
widget.buildIndex();
|
|
assert.true( widget.built );
|
|
assert.deepEqual( widget.index, [] );
|
|
|
|
widget.onListNodeUpdate();
|
|
assert.false( widget.built );
|
|
assert.deepEqual( widget.index, [] );
|
|
} );
|
|
|
|
QUnit.test( 'buildSearchIndex when empty', function ( assert ) {
|
|
const widget = new ve.ui.MWReferenceSearchWidget();
|
|
widget.internalList = { getNodeGroups: () => ( {} ) };
|
|
|
|
const index = widget.buildSearchIndex();
|
|
assert.deepEqual( index, [] );
|
|
} );
|
|
|
|
QUnit.test( 'buildSearchIndex with a placeholder', function ( assert ) {
|
|
const widget = new ve.ui.MWReferenceSearchWidget();
|
|
const placeholder = true;
|
|
const node = { getAttribute: () => placeholder };
|
|
const groups = { 'mwReference/': { indexOrder: [ 0 ], firstNodes: [ node ] } };
|
|
widget.internalList = { getNodeGroups: () => groups, getItemNode: () => [] };
|
|
|
|
const index = widget.buildSearchIndex();
|
|
assert.deepEqual( index, [] );
|
|
} );
|
|
|
|
QUnit.test( 'buildSearchIndex', function ( assert ) {
|
|
const widget = new ve.ui.MWReferenceSearchWidget();
|
|
|
|
// XXX: This is a regression test with a fragile setup. Please feel free to delete this test
|
|
// when you feel like it doesn't make sense to update it.
|
|
const placeholder = false;
|
|
const node = {
|
|
getDocument: () => ( {
|
|
getInternalList: () => null
|
|
} ),
|
|
getAttributes: () => ( { listKey: 'literal/foo' } ),
|
|
getAttribute: () => placeholder
|
|
};
|
|
const groups = { 'mwReference/': { indexOrder: [ 0 ], firstNodes: [ node ] } };
|
|
widget.internalList = { getNodeGroups: () => groups, getItemNode: () => [] };
|
|
|
|
const index = widget.buildSearchIndex();
|
|
assert.deepEqual( index.length, 1 );
|
|
assert.deepEqual( index[ 0 ].citation, '1' );
|
|
assert.deepEqual( index[ 0 ].name, 'foo' );
|
|
assert.deepEqual( index[ 0 ].text, '1 foo' );
|
|
} );
|
|
|
|
QUnit.test( 'isIndexEmpty', function ( assert ) {
|
|
const widget = new ve.ui.MWReferenceSearchWidget();
|
|
assert.true( widget.isIndexEmpty() );
|
|
|
|
// XXX: This is a regression test with a fragile setup. Please feel free to delete this test
|
|
// when you feel like it doesn't make sense to update it.
|
|
const internalList = {
|
|
connect: () => null,
|
|
getListNode: () => ( { connect: () => null } ),
|
|
getNodeGroups: () => ( { 'mwReference/': { indexOrder: [ 0 ] } } )
|
|
};
|
|
widget.setInternalList( internalList );
|
|
assert.false( widget.isIndexEmpty() );
|
|
} );
|
|
|
|
QUnit.test( 'buildSearchResults', function ( assert ) {
|
|
const widget = new ve.ui.MWReferenceSearchWidget();
|
|
widget.index = [ { text: 'a', reference: 'model-a' }, { text: 'b' } ];
|
|
|
|
assert.strictEqual( widget.getResults().getItemCount(), 0 );
|
|
const results = widget.buildSearchResults( 'A' );
|
|
assert.strictEqual( results.length, 1 );
|
|
assert.strictEqual( results[ 0 ].getData(), 'model-a' );
|
|
} );
|