mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-11-24 06:54:00 +00:00
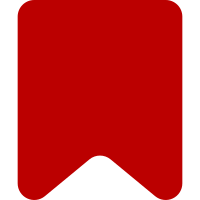
There is the patch(I4297aea3489bb66c98c664da2332584c27793bfa) which will add DeprecationHelper trait to Parser class in order to deprecate public Parser::mUser. DeprecationHelper trait has appropriate magic methods which help to use dynamic properties. In order not to mock them via createMock(), so getMockBuilder() and onlyMethods() was used. onlyMethods() method helps to specify methods which need to be mocked. Now we can use dynamic properties in Parser related tests of Cite extension. Bug: T285713 Change-Id: Ie75c9cd66d296ce7cf15432e2093817e18004443
99 lines
2.4 KiB
PHP
99 lines
2.4 KiB
PHP
<?php
|
|
|
|
namespace Cite\Tests\Unit;
|
|
|
|
use Cite\Cite;
|
|
use Cite\Hooks\CiteParserHooks;
|
|
use Parser;
|
|
use ParserOptions;
|
|
use ParserOutput;
|
|
use StripState;
|
|
|
|
/**
|
|
* @coversDefaultClass \Cite\Hooks\CiteParserHooks
|
|
*
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class CiteParserHooksTest extends \MediaWikiUnitTestCase {
|
|
|
|
/**
|
|
* @covers ::onParserFirstCallInit
|
|
*/
|
|
public function testOnParserFirstCallInit() {
|
|
$parser = $this->createMock( Parser::class );
|
|
$parser->expects( $this->exactly( 2 ) )
|
|
->method( 'setHook' )
|
|
->withConsecutive(
|
|
[ 'ref', $this->isType( 'callable' ) ],
|
|
[ 'references', $this->isType( 'callable' ) ]
|
|
);
|
|
|
|
$citeParserHooks = new CiteParserHooks();
|
|
$citeParserHooks->onParserFirstCallInit( $parser );
|
|
}
|
|
|
|
/**
|
|
* @covers ::onParserClearState
|
|
*/
|
|
public function testOnParserClearState() {
|
|
$parser = $this->createParser();
|
|
$parser->extCite = $this->createMock( Cite::class );
|
|
|
|
$citeParserHooks = new CiteParserHooks();
|
|
/** @var Parser $parser */
|
|
$citeParserHooks->onParserClearState( $parser );
|
|
|
|
$this->assertFalse( isset( $parser->extCite ) );
|
|
}
|
|
|
|
/**
|
|
* @covers ::onParserCloned
|
|
*/
|
|
public function testOnParserCloned() {
|
|
$parser = $this->createParser();
|
|
$parser->extCite = $this->createMock( Cite::class );
|
|
|
|
$citeParserHooks = new CiteParserHooks();
|
|
/** @var Parser $parser */
|
|
$citeParserHooks->onParserCloned( $parser );
|
|
|
|
$this->assertFalse( isset( $parser->extCite ) );
|
|
}
|
|
|
|
/**
|
|
* @covers ::onParserAfterParse
|
|
*/
|
|
public function testAfterParseHooks() {
|
|
$cite = $this->createMock( Cite::class );
|
|
$cite->expects( $this->once() )
|
|
->method( 'checkRefsNoReferences' );
|
|
|
|
$parserOptions = $this->createMock( ParserOptions::class );
|
|
$parserOptions->method( 'getIsSectionPreview' )
|
|
->willReturn( false );
|
|
|
|
$parser = $this->createParser( [ 'getOptions', 'getOutput' ] );
|
|
$parser->method( 'getOptions' )
|
|
->willReturn( $parserOptions );
|
|
$parser->method( 'getOutput' )
|
|
->willReturn( $this->createMock( ParserOutput::class ) );
|
|
$parser->extCite = $cite;
|
|
|
|
$text = '';
|
|
$citeParserHooks = new CiteParserHooks();
|
|
$citeParserHooks->onParserAfterParse( $parser, $text, $this->createMock( StripState::class ) );
|
|
}
|
|
|
|
/**
|
|
* @param array $configurableMethods
|
|
* @return Parser
|
|
*/
|
|
private function createParser( $configurableMethods = [] ) {
|
|
return $this->getMockBuilder( Parser::class )
|
|
->disableOriginalConstructor()
|
|
->onlyMethods( $configurableMethods )
|
|
->getMock();
|
|
}
|
|
|
|
}
|