mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-12-18 09:40:49 +00:00
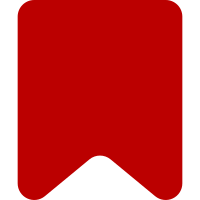
It's a feature of named refs that we only know at the time of inserting
the references list whether they have content or not, and are therefore
in err. The strategy of 4438a72
was to keep pointers to all named ref
nodes so that if an error does occur, we can mark them up.
The problem with embedded content is that, at the time when we find out
about the errors, it's been serialized and stored, and so any pointers
we might have kept around are no longer live or relevant. We need to go
back and process all that embedded content again to find where the refs
with errors are hiding.
This patch slightly optimizes that by keeping a map of all the errors
for refs in embedded content so that only one pass is necessary, rather
than for each references list. Also note that, in the common case, this
pass won't run since we won't have any errors in embedded content.
Bug: T266356
Change-Id: I32e7bfa796cd4382c43b3b1d17b925dc97ce9f7f
47 lines
1.1 KiB
PHP
47 lines
1.1 KiB
PHP
<?php
|
|
declare( strict_types = 1 );
|
|
|
|
namespace Wikimedia\Parsoid\Ext\Cite;
|
|
|
|
use DOMElement;
|
|
use DOMNode;
|
|
use Wikimedia\Parsoid\Ext\DOMProcessor;
|
|
use Wikimedia\Parsoid\Ext\ParsoidExtensionAPI;
|
|
|
|
/**
|
|
* wt -> html DOM PostProcessor
|
|
*/
|
|
class RefProcessor extends DOMProcessor {
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function wtPostprocess(
|
|
ParsoidExtensionAPI $extApi, DOMNode $node, array $options, bool $atTopLevel
|
|
): void {
|
|
if ( $atTopLevel ) {
|
|
$refsData = new ReferencesData();
|
|
References::processRefs( $extApi, $refsData, $node );
|
|
References::insertMissingReferencesIntoDOM( $extApi, $refsData, $node );
|
|
if ( count( $refsData->embeddedErrors ) > 0 ) {
|
|
References::addEmbeddedErrors( $extApi, $refsData, $node );
|
|
}
|
|
}
|
|
}
|
|
|
|
/**
|
|
* html -> wt DOM PreProcessor
|
|
*
|
|
* Nothing to do right now.
|
|
*
|
|
* But, for example, as part of some future functionality, this could be used to
|
|
* reconstitute page-level information from local annotations left behind by editing clients.
|
|
*
|
|
* @param ParsoidExtensionAPI $extApi
|
|
* @param DOMElement $root
|
|
*/
|
|
public function htmlPreprocess( ParsoidExtensionAPI $extApi, DOMElement $root ): void {
|
|
// TODO
|
|
}
|
|
}
|