mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-11-23 22:45:20 +00:00
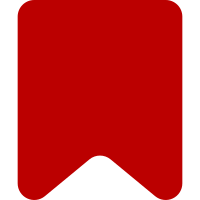
I believe this makes the code less brittle, and also makes it a bit more obvious what these strings are meant to represent. Change-Id: I0c5cdaa0b94b525ad3e65278ca9bf088f480df40
74 lines
1.9 KiB
PHP
74 lines
1.9 KiB
PHP
<?php
|
|
|
|
namespace Cite\Tests\Integration;
|
|
|
|
use Cite\AnchorFormatter;
|
|
use MediaWiki\MainConfigNames;
|
|
use MediaWiki\Parser\Sanitizer;
|
|
use Wikimedia\TestingAccessWrapper;
|
|
|
|
/**
|
|
* @covers \Cite\AnchorFormatter
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class AnchorFormatterTest extends \MediaWikiIntegrationTestCase {
|
|
|
|
protected function setUp(): void {
|
|
parent::setUp();
|
|
$this->overrideConfigValue( MainConfigNames::FragmentMode, [ 'html5' ] );
|
|
}
|
|
|
|
public function testRefKey() {
|
|
$formatter = new AnchorFormatter();
|
|
|
|
$this->assertSame(
|
|
'cite_ref-key',
|
|
$formatter->backLink( 'key', null ) );
|
|
$this->assertSame(
|
|
'cite_ref-key_2',
|
|
$formatter->backLink( 'key', '2' ) );
|
|
}
|
|
|
|
public function testGetReferencesKey() {
|
|
$formatter = new AnchorFormatter();
|
|
|
|
$this->assertSame(
|
|
'cite_note-key',
|
|
$formatter->jumpLink( 'key' ) );
|
|
}
|
|
|
|
/**
|
|
* @dataProvider provideKeyNormalizations
|
|
*/
|
|
public function testNormalizeKey( $key, $expected ) {
|
|
/** @var AnchorFormatter $formatter */
|
|
$formatter = TestingAccessWrapper::newFromObject( new AnchorFormatter() );
|
|
$normalized = $formatter->normalizeKey( $key );
|
|
$encoded = Sanitizer::safeEncodeAttribute( Sanitizer::escapeIdForLink( $normalized ) );
|
|
$this->assertSame( $expected, $encoded );
|
|
}
|
|
|
|
public static function provideKeyNormalizations() {
|
|
return [
|
|
[ 'a b', 'a_b' ],
|
|
[ 'a __ b', 'a_b' ],
|
|
[ ':', ':' ],
|
|
[ "\t\n", '_' ],
|
|
[ "'", ''' ],
|
|
[ "''", '''' ],
|
|
[ '"%&/<>?[]{|}', '"%&/<>?[]{|}' ],
|
|
[ 'ISBN', 'ISBN' ],
|
|
|
|
[ 'nature%20phylo', 'nature%2520phylo' ],
|
|
[ 'Mininova%2E26%2E11%2E2009', 'Mininova%252E26%252E11%252E2009' ],
|
|
[ '%c8%98tiri_2019', '%25c8%2598tiri_2019' ],
|
|
[ 'play%21', 'play%2521' ],
|
|
[ 'Terry+O%26rsquo%3BN…</ref', 'Terry+O%2526rsquo%253BN…</ref' ],
|
|
[ '9 pm', '9&nbsp;pm' ],
|
|
[ 'n%25%32%30n', 'n%2525%2532%2530n' ],
|
|
[ 'a_ %20a', 'a_%2520a' ],
|
|
];
|
|
}
|
|
|
|
}
|