mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-11-12 01:01:29 +00:00
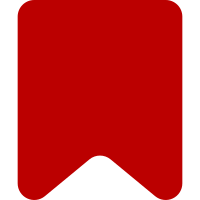
This patch does two things: * Add strict PHP 7 type hints to most code. * Narrow the interface of the checkRefsNoReferences() method to not require a ParserOptions object any more. Change-Id: I91c6a2d9b76915d7677a3f735ee8e054c898fcc5
56 lines
1.3 KiB
PHP
56 lines
1.3 KiB
PHP
<?php
|
|
|
|
namespace Cite\Tests;
|
|
|
|
use Cite\Cite;
|
|
use Language;
|
|
use Parser;
|
|
use ParserOptions;
|
|
use ParserOutput;
|
|
use StripState;
|
|
|
|
/**
|
|
* @coversDefaultClass \Cite\Cite
|
|
*
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class CiteTest extends \MediaWikiIntegrationTestCase {
|
|
|
|
protected function setUp() : void {
|
|
parent::setUp();
|
|
|
|
$this->setMwGlobals( [
|
|
'wgCiteBookReferencing' => true,
|
|
] );
|
|
}
|
|
|
|
/**
|
|
* @covers ::guardedRef
|
|
*/
|
|
public function testBookExtendsPageProperty() {
|
|
$mockOutput = $this->createMock( ParserOutput::class );
|
|
$mockOutput->expects( $this->once() )
|
|
->method( 'setProperty' )
|
|
->with( Cite::BOOK_REF_PROPERTY, true );
|
|
|
|
$parserOptions = $this->createMock( ParserOptions::class );
|
|
$parserOptions->method( 'getUserLangObj' )
|
|
->willReturn( $this->createMock( Language::class ) );
|
|
|
|
$mockParser = $this->createMock( Parser::class );
|
|
$mockParser->method( 'recursiveTagParse' )
|
|
->willReturn( '' );
|
|
$mockParser->method( 'getOptions' )
|
|
->willReturn( $parserOptions );
|
|
$mockParser->method( 'getOutput' )
|
|
->willReturn( $mockOutput );
|
|
$mockParser->method( 'getStripState' )
|
|
->willReturn( $this->createMock( StripState::class ) );
|
|
|
|
$cite = new Cite();
|
|
$cite->ref( 'contentA', [ 'name' => 'a' ], $mockParser );
|
|
$cite->ref( 'contentB', [ Cite::BOOK_REF_ATTRIBUTE => 'a' ], $mockParser );
|
|
}
|
|
|
|
}
|