mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-12-02 18:46:18 +00:00
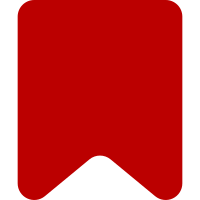
Make sure that we're waiting for the page creation to finish, and assert that the API request was successful. This is mostly a diagnostic improvement to help debug flaky tests. Bug: T355602 Change-Id: I398cffa724e9b6d733df46676478cc98dccd884d
87 lines
3.3 KiB
JavaScript
87 lines
3.3 KiB
JavaScript
import * as helpers from '../utils/functions.helper.js';
|
|
|
|
const title = getTestString( 'CiteTest-title' );
|
|
const encodedTitle = encodeURIComponent( title );
|
|
|
|
function getTestString( prefix = '' ) {
|
|
return prefix + Math.random().toString();
|
|
}
|
|
|
|
describe( 'Cite backlinks test', () => {
|
|
before( () => {
|
|
cy.visit( '/index.php' );
|
|
|
|
const wikiText = 'This is reference #1: <ref name="a">This is citation #1 for reference #1 and #2</ref><br> ' +
|
|
'This is reference #2: <ref name="a" /><br>' +
|
|
'This is reference #3: <ref>This is citation #2</ref><br>' +
|
|
'<references />';
|
|
|
|
// Rely on the retry behavior of Cypress assertions to use this as a "wait"
|
|
// until the specified conditions are met.
|
|
cy.window().should( 'have.property', 'mw' ).and( 'have.property', 'loader' ).and( 'have.property', 'using' );
|
|
cy.window().then( async ( win ) => {
|
|
await win.mw.loader.using( 'mediawiki.api' );
|
|
const response = await new win.mw.Api().create( title, {}, wikiText );
|
|
expect( response.result ).to.equal( 'Success' );
|
|
} );
|
|
} );
|
|
|
|
beforeEach( () => {
|
|
cy.visit( `/index.php?title=${ encodedTitle }` );
|
|
|
|
cy.window().should( 'have.property', 'mw' ).and( 'have.property', 'loader' ).and( 'have.property', 'using' );
|
|
cy.window().then( async ( win ) => {
|
|
await win.mw.loader.using( 'mediawiki.base' ).then( async function () {
|
|
await win.mw.hook( 'wikipage.content' ).add( function () {} );
|
|
} );
|
|
} );
|
|
} );
|
|
|
|
it( 'hides clickable up arrow by default when there are multiple backlinks', () => {
|
|
helpers.getCiteMultiBacklink( 1 ).should( 'not.exist' );
|
|
} );
|
|
|
|
it( 'hides clickable up arrow when jumping back from multiple used references', () => {
|
|
helpers.getReference( 2 ).click();
|
|
helpers.getCiteMultiBacklink( 1 ).click();
|
|
// The up-pointing arrow in the reference line is not linked
|
|
helpers.getCiteMultiBacklink( 1 ).should( 'not.be.visible' );
|
|
} );
|
|
|
|
it( 'shows clickable up arrow when jumping to multiple used references', () => {
|
|
helpers.getReference( 2 ).click();
|
|
helpers.getCiteMultiBacklink( 1 ).should( 'be.visible' );
|
|
|
|
helpers.getFragmentFromLink( helpers.getCiteMultiBacklink( 1 ) ).then( ( linkFragment ) => {
|
|
helpers.getReference( 2 ).invoke( 'attr', 'id' ).should( 'eq', linkFragment );
|
|
} );
|
|
} );
|
|
|
|
it( 'highlights backlink in the reference list for the clicked reference when there are multiple used references', () => {
|
|
cy.get( '.mw-page-title-main' ).should( 'be.visible' );
|
|
helpers.getReference( 2 ).click();
|
|
helpers.getCiteSubBacklink( 2 ).should( 'have.class', 'mw-cite-targeted-backlink' );
|
|
} );
|
|
|
|
it( 'uses the last clicked target for the clickable up arrow on multiple used references', () => {
|
|
helpers.getReference( 2 ).click();
|
|
helpers.getReference( 1 ).click();
|
|
|
|
helpers.getFragmentFromLink( helpers.getCiteMultiBacklink( 1 ) ).then( ( linkFragment ) => {
|
|
helpers.getReference( 1 ).invoke( 'attr', 'id' ).then( ( referenceId ) => {
|
|
expect( linkFragment ).to.equal( referenceId );
|
|
|
|
} );
|
|
} );
|
|
} );
|
|
|
|
it( 'retains backlink visibility for unnamed references when interacting with other references', () => {
|
|
helpers.getReference( 3 ).click();
|
|
helpers.getCiteSingleBacklink( 2 ).click();
|
|
// Doesn' matter what is focused next, just needs to be something else
|
|
helpers.getReference( 1 ).click();
|
|
// The backlink of the unnamed reference is still visible
|
|
helpers.getCiteSingleBacklink( 2 ).should( 'be.visible' );
|
|
} );
|
|
} );
|