mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-11-13 17:53:09 +00:00
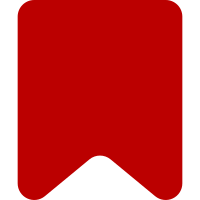
There are multiple formats for these IDs: cite_ref-1, cite_ref-2, and so on for anonymous <ref>s without a name. cite_ref-name_1-0 for named references, where "name" is the custom name, and "_1" is the sequential number for the reference (same number as above). The final "-0" is counting the usages. If a named <ref> is only used once, there is no cite_ref-name_1-1 anywhere on the page. The later was already checked by the code. But we forgot about unnamed references! As a consequence IDs like the cite_ref-1 above got misdetected as reused references. This patch tries hard to extract code into named functions, so it becomes much more clear what they do, and why. Bug: T215317 Change-Id: Iedb5b0c3dffae19bad7df9a43ed2d4512b3921ec
119 lines
3.4 KiB
JavaScript
119 lines
3.4 KiB
JavaScript
/**
|
|
* @author Thiemo Kreuz
|
|
*/
|
|
( function () {
|
|
'use strict';
|
|
|
|
/**
|
|
* Checks if the ID uses a composite format that does not only consist of a sequential number,
|
|
* as specified in "cite_reference_link_key_with_num".
|
|
*
|
|
* @param {string} id
|
|
* @return {boolean}
|
|
*/
|
|
function isNamedReference( id ) {
|
|
var prefix = mw.msg( 'cite_reference_link_prefix' );
|
|
|
|
// Note: This assumes IDs start with the prefix; this is guaranteed by the parser function
|
|
return /\D/.test( id.slice( prefix.length ) );
|
|
}
|
|
|
|
/**
|
|
* @param {string} id
|
|
* @param {jQuery} $content
|
|
* @return {boolean}
|
|
*/
|
|
function isReusedNamedReference( id, $content ) {
|
|
if ( !isNamedReference( id ) ) {
|
|
return false;
|
|
}
|
|
|
|
// Either the ID is already a reuse, or at least one reuse exists somewhere else on the page
|
|
return id.slice( -2 ) !== '-0' ||
|
|
$content.find( '.references a[href="#' + $.escapeSelector( id.slice( 0, -1 ) ) + '1"]' ).length;
|
|
}
|
|
|
|
/**
|
|
* @param {jQuery} $backlinkWrapper
|
|
* @return {jQuery}
|
|
*/
|
|
function makeUpArrowLink( $backlinkWrapper ) {
|
|
var upArrow,
|
|
textNode = $backlinkWrapper[ 0 ].firstChild,
|
|
accessibilityLabel = mw.msg( 'cite_references_link_accessibility_back_label' ),
|
|
$upArrowLink = $( '<a>' )
|
|
.addClass( 'mw-cite-up-arrow-backlink' )
|
|
.attr( 'aria-label', accessibilityLabel )
|
|
.attr( 'title', accessibilityLabel );
|
|
|
|
if ( !textNode ) {
|
|
return $upArrowLink;
|
|
}
|
|
|
|
// Skip additional, custom HTML wrappers, if any.
|
|
while ( textNode.firstChild ) {
|
|
textNode = textNode.firstChild;
|
|
}
|
|
|
|
if ( textNode.nodeType !== Node.TEXT_NODE || textNode.data.trim() === '' ) {
|
|
return $upArrowLink;
|
|
}
|
|
|
|
upArrow = textNode.data.trim();
|
|
// The text node typically contains "↑ ", and we need to keep the space.
|
|
textNode.data = textNode.data.replace( upArrow, '' );
|
|
|
|
// Create a plain text and a clickable "↑". CSS :target selectors make sure only
|
|
// one is visible at a time.
|
|
$backlinkWrapper.prepend(
|
|
$( '<span>' )
|
|
.addClass( 'mw-cite-up-arrow' )
|
|
.text( upArrow ),
|
|
$upArrowLink
|
|
.text( upArrow )
|
|
);
|
|
|
|
return $upArrowLink;
|
|
}
|
|
|
|
/**
|
|
* @param {jQuery} $backlink
|
|
*/
|
|
function updateUpArrowLink( $backlink ) {
|
|
// It's convenient to stop at the class name, but it's not guaranteed to be there.
|
|
var $backlinkWrapper = $backlink.closest( '.mw-cite-backlink, li' ),
|
|
$upArrowLink = $backlinkWrapper.find( '.mw-cite-up-arrow-backlink' );
|
|
|
|
if ( !$upArrowLink.length && $backlinkWrapper.length ) {
|
|
$upArrowLink = makeUpArrowLink( $backlinkWrapper );
|
|
}
|
|
|
|
$upArrowLink.attr( 'href', $backlink.attr( 'href' ) );
|
|
}
|
|
|
|
mw.hook( 'wikipage.content' ).add( function ( $content ) {
|
|
// We are going to use the ID in the code below, so better be sure one is there.
|
|
$content.find( '.reference[id] > a' ).on( 'click', function () {
|
|
var $backlink,
|
|
id = $( this ).parent().attr( 'id' ),
|
|
className = 'mw-cite-targeted-backlink';
|
|
|
|
$content.find( '.' + className ).removeClass( className );
|
|
|
|
// Bail out if there is not at least a second backlink ("cite_references_link_many").
|
|
if ( !isReusedNamedReference( id, $content ) ) {
|
|
return;
|
|
}
|
|
|
|
// The :not() skips the duplicate link created below. Relevant when double clicking.
|
|
$backlink = $content.find( '.references a[href="#' + $.escapeSelector( id ) + '"]:not(.mw-cite-up-arrow-backlink)' )
|
|
.first()
|
|
.addClass( className );
|
|
|
|
if ( $backlink.length ) {
|
|
updateUpArrowLink( $backlink );
|
|
}
|
|
} );
|
|
} );
|
|
}() );
|