mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2024-09-23 10:20:12 +00:00
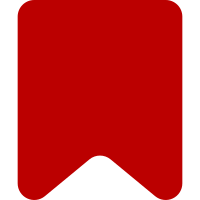
* (bug 6667) Adding nl localisation for Makesysop * (bug 6669) Adding nl localisation for Newuserlog * Fixing notice in Special:Cite.
154 lines
3.5 KiB
PHP
154 lines
3.5 KiB
PHP
<?php
|
|
if (!defined('MEDIAWIKI')) die();
|
|
|
|
global $wgMessageCache, $wgContLang, $wgContLanguageCode, $wgCiteDefaultText;
|
|
|
|
$dir = dirname( __FILE__ ) . DIRECTORY_SEPARATOR;
|
|
$code = $wgContLang->lc( $wgContLanguageCode );
|
|
$file = file_exists( "${dir}cite_text-$code" ) ? "${dir}cite_text-$code" : "${dir}cite_text";
|
|
$wgCiteDefaultText = file_get_contents( $file );
|
|
|
|
class SpecialCite extends SpecialPage {
|
|
function SpecialCite() {
|
|
SpecialPage::SpecialPage( 'Cite' );
|
|
}
|
|
|
|
function execute( $par ) {
|
|
global $wgOut, $wgRequest, $wgUseTidy;
|
|
|
|
// Having tidy on causes whitespace and <pre> tags to
|
|
// be generated around the output of the CiteOutput
|
|
// class TODO FIXME.
|
|
$wgUseTidy = false;
|
|
|
|
$this->setHeaders();
|
|
$this->outputHeader();
|
|
|
|
$page = isset( $par ) ? $par : $wgRequest->getText( 'page' );
|
|
$id = $wgRequest->getInt( 'id' );
|
|
|
|
$title = Title::newFromText( $page );
|
|
$article = new Article( $title );
|
|
|
|
$cform = new CiteForm( $title );
|
|
|
|
if ( is_null( $title ) || ! $article->exists() )
|
|
$cform->execute();
|
|
else {
|
|
$cform->execute();
|
|
|
|
$cout = new CiteOutput( $title, $article, $id );
|
|
$cout->execute();
|
|
}
|
|
}
|
|
}
|
|
|
|
class CiteForm {
|
|
var $mTitle;
|
|
|
|
function CiteForm( &$title ) {
|
|
$this->mTitle =& $title;
|
|
}
|
|
|
|
function execute() {
|
|
global $wgOut, $wgTitle;
|
|
|
|
$wgOut->addHTML(
|
|
wfElement( 'form',
|
|
array(
|
|
'id' => 'specialcite',
|
|
'method' => 'get',
|
|
'action' => $wgTitle->escapeLocalUrl()
|
|
),
|
|
null
|
|
) .
|
|
wfOpenElement( 'label' ) .
|
|
wfMsgHtml( 'cite_page' ) . ' ' .
|
|
wfElement( 'input',
|
|
array(
|
|
'type' => 'text',
|
|
'size' => 20,
|
|
'name' => 'page',
|
|
'value' => is_object( $this->mTitle ) ? $this->mTitle->getPrefixedText() : ''
|
|
),
|
|
''
|
|
) .
|
|
' ' .
|
|
wfElement( 'input',
|
|
array(
|
|
'type' => 'submit',
|
|
'value' => wfMsgHtml( 'cite_submit' )
|
|
),
|
|
''
|
|
) .
|
|
wfCloseElement( 'label' ) .
|
|
wfCloseElement( 'form' )
|
|
);
|
|
}
|
|
|
|
}
|
|
|
|
class CiteOutput {
|
|
var $mTitle, $mArticle, $mId;
|
|
var $mParser, $mParserOptions;
|
|
|
|
function CiteOutput( &$title, &$article, $id ) {
|
|
global $wgHooks, $wgParser;
|
|
|
|
$this->mTitle =& $title;
|
|
$this->mArticle =& $article;
|
|
$this->mId = $id;
|
|
|
|
$wgHooks['ParserGetVariableValueVarCache'][] = array( $this, 'varCache' );
|
|
|
|
$this->genParserOptions();
|
|
$this->genParser();
|
|
|
|
$wgParser->setHook( 'citation', array( $this, 'CiteParse' ) );
|
|
}
|
|
|
|
function execute() {
|
|
global $wgOut, $wgUser, $wgParser, $wgHooks, $wgCiteDefaultText;
|
|
|
|
$wgHooks['ParserGetVariableValueTs'][] = array( $this, 'timestamp' );
|
|
|
|
$msg = wfMsgForContentNoTrans( 'cite_text' );
|
|
if( $msg == '' ) {
|
|
$msg = $wgCiteDefaultText;
|
|
}
|
|
$this->mArticle->fetchContent( $this->mId, false );
|
|
$ret = $wgParser->parse( $msg, $this->mTitle, $this->mParserOptions, false, true, $this->mArticle->getRevIdFetched() );
|
|
$wgOut->addHtml( $ret->getText() );
|
|
}
|
|
|
|
function genParserOptions() {
|
|
global $wgUser;
|
|
$this->mParserOptions = ParserOptions::newFromUser( $wgUser );
|
|
$this->mParserOptions->setDateFormat( MW_DATE_DEFAULT );
|
|
$this->mParserOptions->setEditSection( false );
|
|
}
|
|
|
|
function genParser() {
|
|
$this->mParser = new Parser;
|
|
}
|
|
|
|
function CiteParse( $in, $argv ) {
|
|
global $wgTitle;
|
|
|
|
$ret = $this->mParser->parse( $in, $wgTitle, $this->mParserOptions, false );
|
|
|
|
return $ret->getText();
|
|
}
|
|
|
|
function varCache() { return false; }
|
|
|
|
function timestamp( &$parser, &$ts ) {
|
|
if ( isset( $parser->mTagHooks['citation'] ) )
|
|
$ts = wfTimestamp( TS_UNIX, $this->mArticle->getTimestamp() );
|
|
|
|
return true;
|
|
}
|
|
}
|
|
|
|
?>
|