mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Cite
synced 2025-01-02 08:45:10 +00:00
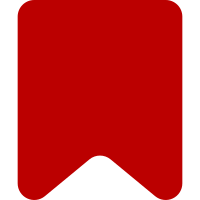
* All classes are in a Cite\ namespace now. No need to repeat the word "Cite" all over the place. * The "key formatter" is more an ID or anchor formatter. The strings it returns are all used in id="…" attributes, as well as in href="#…" links to jump to these IDs. * This patch also removes quite a bunch of callbacks from tests that don't need to be callbacks. * I'm also replacing all json_encode(). * To make the test code more readable, I shorten a bunch of variable names to e.g. $msg. The fact they are mocks is still relevant, and still visible because these variable names are only used in very short scopes. Change-Id: I2bd7c731efd815bcdc5d33bccb0c8e280d55bd06
75 lines
2 KiB
PHP
75 lines
2 KiB
PHP
<?php
|
|
|
|
namespace Cite;
|
|
|
|
use Sanitizer;
|
|
|
|
/**
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class AnchorFormatter {
|
|
|
|
/**
|
|
* @var ReferenceMessageLocalizer
|
|
*/
|
|
private $messageLocalizer;
|
|
|
|
/**
|
|
* @param ReferenceMessageLocalizer $messageLocalizer
|
|
*/
|
|
public function __construct( ReferenceMessageLocalizer $messageLocalizer ) {
|
|
$this->messageLocalizer = $messageLocalizer;
|
|
}
|
|
|
|
/**
|
|
* Return an id for use in wikitext output based on a key and
|
|
* optionally the number of it, used in <references>, not <ref>
|
|
* (since otherwise it would link to itself)
|
|
*
|
|
* @param string $key
|
|
* @param string|null $num The number of the key
|
|
* @return string A key for use in wikitext
|
|
*/
|
|
public function refKey( string $key, string $num = null ) : string {
|
|
// FIXME: Move the message to 'cite_ref_link_*'
|
|
$prefix = $this->messageLocalizer->msg( 'cite_reference_link_prefix' )->text();
|
|
$suffix = $this->messageLocalizer->msg( 'cite_reference_link_suffix' )->text();
|
|
if ( $num !== null ) {
|
|
$key = $this->messageLocalizer->msg( 'cite_reference_link_key_with_num', $key, $num )
|
|
->plain();
|
|
}
|
|
|
|
return $this->normalizeKey( $prefix . $key . $suffix );
|
|
}
|
|
|
|
/**
|
|
* Return an id for use in wikitext output based on a key and
|
|
* optionally the number of it, used in <ref>, not <references>
|
|
* (since otherwise it would link to itself)
|
|
*
|
|
* @param string $key
|
|
* @return string A key for use in wikitext
|
|
*/
|
|
public function getReferencesKey( string $key ) : string {
|
|
$prefix = $this->messageLocalizer->msg( 'cite_references_link_prefix' )->text();
|
|
$suffix = $this->messageLocalizer->msg( 'cite_references_link_suffix' )->text();
|
|
|
|
return $this->normalizeKey( $prefix . $key . $suffix );
|
|
}
|
|
|
|
/**
|
|
* Normalizes and sanitizes a reference key
|
|
*
|
|
* @param string $key
|
|
* @return string
|
|
*/
|
|
private function normalizeKey( string $key ) : string {
|
|
$ret = Sanitizer::escapeIdForAttribute( $key );
|
|
$ret = preg_replace( '/__+/', '_', $ret );
|
|
$ret = Sanitizer::safeEncodeAttribute( $ret );
|
|
|
|
return $ret;
|
|
}
|
|
|
|
}
|