mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CategoryTree
synced 2024-11-27 17:51:00 +00:00
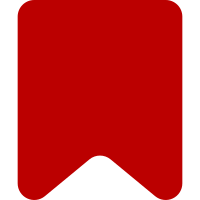
This converts the CategtoryTree extension to the new (MW1.35) hook system. The patch was written live during a hackathon workshop. A recording of the workshop is available at <https://www.youtube.com/watch?v=ZOj44Rbh0tM&t>. Re-applying after fixing: premature access to $wgRequest Restores change: Ie52c393af378a980a2dac4ae7076fd6c016a8e0e Reverts revert: Ieee300c7b35b7069bd7e781610c915f2ecae1bf1 NOTE: the "mode" parameter for category pages seems to be broken, as the mode is not proeprly propoagated to dynamic requests. This was already the case before this change, and this change does not try to fix that issue. I was tempted to just remove the feature entirely, but T137812 inidcates that it may be useful to some. I will comment there to see if this should be fiexed or removed. Bug: T282110 Bug: T271011 Change-Id: Ic3ee05e9dc382f4ada5cfeb9ff5d9a69249cc60d
95 lines
2.4 KiB
PHP
95 lines
2.4 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\CategoryTree;
|
|
|
|
use Category;
|
|
use CategoryViewer;
|
|
|
|
class CategoryTreeCategoryViewer extends CategoryViewer {
|
|
public $child_cats;
|
|
|
|
/**
|
|
* @var CategoryTree
|
|
*/
|
|
public $categorytree;
|
|
|
|
/**
|
|
* @return CategoryTree
|
|
*/
|
|
private function getCategoryTree() {
|
|
if ( !isset( $this->categorytree ) ) {
|
|
if ( !Hooks::shouldForceHeaders() ) {
|
|
CategoryTree::setHeaders( $this->getOutput() );
|
|
}
|
|
|
|
$options = $this->getConfig()->get( 'CategoryTreeCategoryPageOptions' );
|
|
|
|
$mode = $this->getRequest()->getVal( 'mode' );
|
|
if ( $mode !== null ) {
|
|
$options['mode'] = CategoryTree::decodeMode( $mode );
|
|
}
|
|
|
|
$this->categorytree = new CategoryTree( $options );
|
|
}
|
|
|
|
return $this->categorytree;
|
|
}
|
|
|
|
/**
|
|
* Add a subcategory to the internal lists
|
|
* @param Category $cat
|
|
* @param string $sortkey
|
|
* @param int $pageLength
|
|
*/
|
|
public function addSubcategoryObject( Category $cat, $sortkey, $pageLength ) {
|
|
$title = $cat->getTitle();
|
|
|
|
if ( $this->getRequest()->getCheck( 'notree' ) ) {
|
|
parent::addSubcategoryObject( $cat, $sortkey, $pageLength );
|
|
return;
|
|
}
|
|
|
|
$tree = $this->getCategoryTree();
|
|
|
|
$this->children[] = $tree->renderNodeInfo( $title, $cat );
|
|
|
|
$this->children_start_char[] = $this->getSubcategorySortChar( $title, $sortkey );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function clearCategoryState() {
|
|
$this->child_cats = [];
|
|
parent::clearCategoryState();
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function finaliseCategoryState() {
|
|
if ( $this->flip ) {
|
|
$this->child_cats = array_reverse( $this->child_cats );
|
|
}
|
|
parent::finaliseCategoryState();
|
|
}
|
|
}
|