mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CategoryTree
synced 2024-11-15 12:00:44 +00:00
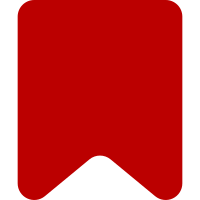
* Implemented memcached caching for ajax requests with invalidation via page_touched * General refactoring, moved some global functions into a class * Lazy initialisation of messages, initialise the <head> section in the output only when a categorytree tag is present. * Eliminated all $wgUser and $wgLang accesses from ajax requests, using message placeholders. * Added speak:none style in .CategoryTreeBullet in case voice browsers try to read out the punctuation
69 lines
2.2 KiB
JavaScript
69 lines
2.2 KiB
JavaScript
/*
|
|
* JavaScript functions for the CategoryTree extension, an AJAX based gadget
|
|
* to display the category structure of a wiki
|
|
*
|
|
* @package MediaWiki
|
|
* @subpackage Extensions
|
|
* @author Daniel Kinzler <duesentrieb@brightbyte.de>
|
|
* @copyright © 2006 Daniel Kinzler
|
|
* @licence GNU General Public Licence 2.0 or later
|
|
*/
|
|
|
|
function categoryTreeNextDiv(e) {
|
|
n= e.nextSibling;
|
|
while ( n && ( n.nodeType != 1 || n.nodeName != 'DIV') ) {
|
|
//alert('nodeType: ' + n.nodeType + '; nodeName: ' + n.nodeName);
|
|
n= n.nextSibling;
|
|
}
|
|
|
|
return n;
|
|
}
|
|
|
|
function categoryTreeExpandNode(cat, mode, lnk) {
|
|
var div= categoryTreeNextDiv( lnk.parentNode.parentNode );
|
|
|
|
div.style.display= 'block';
|
|
lnk.innerHTML= '–';
|
|
lnk.title= categoryTreeCollapseMsg;
|
|
lnk.onclick= function() { categoryTreeCollapseNode(cat, mode, lnk) }
|
|
|
|
if (lnk.className != "CategoryTreeLoaded") {
|
|
categoryTreeLoadNode(cat, mode, lnk, div);
|
|
}
|
|
}
|
|
|
|
function categoryTreeCollapseNode(cat, mode, lnk) {
|
|
var div= categoryTreeNextDiv( lnk.parentNode.parentNode );
|
|
|
|
div.style.display= 'none';
|
|
lnk.innerHTML= '+';
|
|
lnk.title= categoryTreeExpandMsg;
|
|
lnk.onclick= function() { categoryTreeExpandNode(cat, mode, lnk) }
|
|
}
|
|
|
|
function categoryTreeLoadNode(cat, mode, lnk, div) {
|
|
div.style.display= 'block';
|
|
lnk.className= 'CategoryTreeLoaded';
|
|
lnk.innerHTML= '–';
|
|
lnk.title= categoryTreeCollapseMsg;
|
|
lnk.onclick= function() { categoryTreeCollapseNode(cat, mode, lnk) }
|
|
|
|
categoryTreeLoadChildren(cat, mode, div)
|
|
}
|
|
|
|
function categoryTreeLoadChildren(cat, mode, div) {
|
|
div.innerHTML= '<i class="CategoryTreeNotice">' + categoryTreeLoadingMsg + '</i>';
|
|
|
|
function f( result ) {
|
|
if ( result == '' ) result= '<i class="CategoryTreeNotice">' + categoryTreeNothingFoundMsg + '</i>';
|
|
result = result.replace(/##LOAD##/g, categoryTreeLoadMsg);
|
|
div.innerHTML= result;
|
|
}
|
|
|
|
categoryTreeDoCall( cat, mode, f );
|
|
}
|
|
|
|
function categoryTreeDoCall() {
|
|
sajax_do_call( "efCategoryTreeAjaxWrapper", categoryTreeDoCall.arguments );
|
|
}
|