mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/CategoryTree
synced 2024-11-15 03:43:55 +00:00
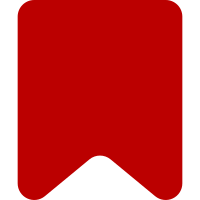
* Add @inheritDoc to functions that are inheritance. * Move comments to a single line. Remove the phpcs rule exceptions: * MediaWiki.Commenting.FunctionComment.MissingDocumentationProtected * MediaWiki.Commenting.FunctionComment.MissingDocumentationPublic * MediaWiki.WhiteSpace.SpaceBeforeSingleLineComment.NewLineComment Change-Id: I742ee19f0a3d2d62e08b6f2e35cd9a6b3940cd22
85 lines
2.1 KiB
PHP
85 lines
2.1 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
class CategoryTreeCategoryViewer extends CategoryViewer {
|
|
public $child_cats;
|
|
|
|
/**
|
|
* @var CategoryTree
|
|
*/
|
|
public $categorytree;
|
|
|
|
/**
|
|
* @return CategoryTree
|
|
*/
|
|
private function getCategoryTree() {
|
|
global $wgCategoryTreeCategoryPageOptions;
|
|
|
|
if ( !isset( $this->categorytree ) ) {
|
|
if ( !CategoryTreeHooks::shouldForceHeaders() ) {
|
|
CategoryTree::setHeaders( $this->getOutput() );
|
|
}
|
|
|
|
$this->categorytree = new CategoryTree( $wgCategoryTreeCategoryPageOptions );
|
|
}
|
|
|
|
return $this->categorytree;
|
|
}
|
|
|
|
/**
|
|
* Add a subcategory to the internal lists
|
|
* @param Category $cat
|
|
* @param string $sortkey
|
|
* @param int $pageLength
|
|
*/
|
|
public function addSubcategoryObject( Category $cat, $sortkey, $pageLength ) {
|
|
$title = $cat->getTitle();
|
|
|
|
if ( $this->getRequest()->getCheck( 'notree' ) ) {
|
|
parent::addSubcategoryObject( $cat, $sortkey, $pageLength );
|
|
return;
|
|
}
|
|
|
|
$tree = $this->getCategoryTree();
|
|
|
|
$this->children[] = $tree->renderNodeInfo( $title, $cat );
|
|
|
|
$this->children_start_char[] = $this->getSubcategorySortChar( $title, $sortkey );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function clearCategoryState() {
|
|
$this->child_cats = [];
|
|
parent::clearCategoryState();
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function finaliseCategoryState() {
|
|
if ( $this->flip ) {
|
|
$this->child_cats = array_reverse( $this->child_cats );
|
|
}
|
|
parent::finaliseCategoryState();
|
|
}
|
|
}
|