mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 10:15:24 +00:00
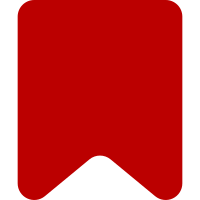
This allows us to: - Defer handling of the block to the main module - Choose the right message depending on the block type - Avoid directly using the apierror-blocked message, which could change in the future. Change-Id: If2e32bd2ccf5e314aa51203afd1522b8481377e0 Follows-up: I35f2c6e701a24dccb6e26e3f3c578fd44f68127d
86 lines
1.8 KiB
PHP
86 lines
1.8 KiB
PHP
<?php
|
|
|
|
class ApiAbuseFilterUnblockAutopromote extends ApiBase {
|
|
/**
|
|
* @see ApiBase::execute()
|
|
*/
|
|
public function execute() {
|
|
$this->checkUserRightsAny( 'abusefilter-modify' );
|
|
|
|
$params = $this->extractRequestParams();
|
|
$target = User::newFromName( $params['user'] );
|
|
|
|
if ( $target === false ) {
|
|
$encParamName = $this->encodeParamName( 'user' );
|
|
$this->dieWithError(
|
|
[ 'apierror-baduser', $encParamName, wfEscapeWikiText( $params['user'] ) ],
|
|
"baduser_{$encParamName}"
|
|
);
|
|
}
|
|
|
|
$block = $this->getUser()->getBlock();
|
|
if ( $block && $block->isSitewide() ) {
|
|
$this->dieBlocked( $block );
|
|
}
|
|
|
|
$msg = $this->msg( 'abusefilter-tools-restoreautopromote' )->inContentLanguage()->text();
|
|
$res = AbuseFilter::unblockAutopromote( $target, $this->getUser(), $msg );
|
|
|
|
if ( $res === false ) {
|
|
$this->dieWithError( [ 'abusefilter-reautoconfirm-none', $target->getName() ], 'notsuspended' );
|
|
}
|
|
|
|
$finalResult = [ 'user' => $params['user'] ];
|
|
$this->getResult()->addValue( null, $this->getModuleName(), $finalResult );
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::mustBePosted()
|
|
* @return bool
|
|
*/
|
|
public function mustBePosted() {
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::isWriteMode()
|
|
* @return bool
|
|
*/
|
|
public function isWriteMode() {
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getAllowedParams()
|
|
* @return array
|
|
*/
|
|
public function getAllowedParams() {
|
|
return [
|
|
'user' => [
|
|
ApiBase::PARAM_TYPE => 'user',
|
|
ApiBase::PARAM_REQUIRED => true
|
|
],
|
|
'token' => null,
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::needsToken()
|
|
* @return string
|
|
*/
|
|
public function needsToken() {
|
|
return 'csrf';
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
* @return array
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
return [
|
|
'action=abusefilterunblockautopromote&user=Example&token=123ABC'
|
|
=> 'apihelp-abusefilterunblockautopromote-example-1',
|
|
];
|
|
}
|
|
}
|