mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-12 00:38:23 +00:00
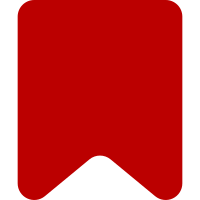
Follows-up I5a5420df13893386. > We lose useful coverage and waste valuable time on keeping tags > accurate through refactors (or worse, forget to do so). > > Tracking tiny per-method details wastes time in realizing (and > fixing) when people inevitably don't keep them in sync, and time > lost in finding uncovered code to write tests to realize it was > already covered but "not yet claimed". > > Given all used methods are de-facto and liberally claimed, and > that we keep the coverage limited to the subject class, this > maintains the spirit and intent. PHPUnit offers a more precise > tool when you need it (i.e. when testing legacy monster classes), > but for well-written code, the class-wide tag suffices. Ref https://gerrit.wikimedia.org/r/q/owner:Krinkle+is:merged+message:Widen Change-Id: If7304d8b5b43ab8a051fbcecced331a787bab960
53 lines
2.3 KiB
PHP
53 lines
2.3 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Extension\AbuseFilter\Hooks\Handlers\SchemaChangesHandler;
|
|
use MediaWiki\User\UserGroupManager;
|
|
|
|
/**
|
|
* @group Database
|
|
* @covers \MediaWiki\Extension\AbuseFilter\Hooks\Handlers\SchemaChangesHandler
|
|
* @todo Make this a unit test once User::newSystemUser is moved to a service
|
|
*/
|
|
class SchemaChangesHandlerTest extends MediaWikiIntegrationTestCase {
|
|
|
|
public function testConstruct() {
|
|
$this->assertInstanceOf(
|
|
SchemaChangesHandler::class,
|
|
new SchemaChangesHandler(
|
|
$this->createMock( MessageLocalizer::class ),
|
|
$this->createMock( UserGroupManager::class )
|
|
)
|
|
);
|
|
}
|
|
|
|
public function testCreateAbuseFilterUser_invalidUserName() {
|
|
$noRowUpdater = $this->createMock( DatabaseUpdater::class );
|
|
$noRowUpdater->method( 'updateRowExists' )->willReturn( false );
|
|
$invalidML = $this->createMock( MessageLocalizer::class );
|
|
$invalidML->method( 'msg' )->with( 'abusefilter-blocker' )->willReturn( $this->getMockMessage( '' ) );
|
|
$handler = new SchemaChangesHandler( $invalidML, $this->createNoOpMock( UserGroupManager::class ) );
|
|
$this->assertFalse( $handler->createAbuseFilterUser( $noRowUpdater ) );
|
|
}
|
|
|
|
public function testCreateAbuseFilterUser_alreadyCreated() {
|
|
$rowExistsUpdater = $this->createMock( DatabaseUpdater::class );
|
|
$rowExistsUpdater->method( 'updateRowExists' )->willReturn( true );
|
|
$validML = $this->createMock( MessageLocalizer::class );
|
|
$validML->method( 'msg' )->with( 'abusefilter-blocker' )->willReturn( $this->getMockMessage( 'Foo' ) );
|
|
$handler = new SchemaChangesHandler( $validML, $this->createNoOpMock( UserGroupManager::class ) );
|
|
$this->assertFalse( $handler->createAbuseFilterUser( $rowExistsUpdater ) );
|
|
}
|
|
|
|
public function testCreateAbuseFilterUser_success() {
|
|
$noRowUpdater = $this->createMock( DatabaseUpdater::class );
|
|
$noRowUpdater->method( 'updateRowExists' )->willReturn( false );
|
|
$validML = $this->createMock( MessageLocalizer::class );
|
|
$validML->method( 'msg' )->with( 'abusefilter-blocker' )->willReturn( $this->getMockMessage( 'Foo' ) );
|
|
$ugm = $this->createMock( UserGroupManager::class );
|
|
$ugm->expects( $this->once() )->method( 'addUserToGroup' );
|
|
$okHandler = new SchemaChangesHandler( $validML, $ugm );
|
|
$this->assertTrue( $okHandler->createAbuseFilterUser( $noRowUpdater ) );
|
|
}
|
|
|
|
}
|