mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 18:19:38 +00:00
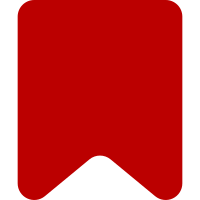
Adding PHPdocs to every class members, in every file. This patch only touches comments, and moved properties on their own lines. Note that some of these properties would need to be moved, somehow changed, or just removed (either because they're old, unused leftovers, or just because we can move them to local scope), but I wanted to keep this patch doc-only. Change-Id: I9fe701445bea8f09d82783789ff1ec537ac6704b
90 lines
2 KiB
PHP
90 lines
2 KiB
PHP
<?php
|
|
|
|
class AbuseFilterExaminePager extends ReverseChronologicalPager {
|
|
/**
|
|
* @var AbuseFilterChangesList Our changes list
|
|
*/
|
|
public $mChangesList;
|
|
/**
|
|
* @var AbuseFilterViewExamine The associated view
|
|
*/
|
|
public $mPage;
|
|
|
|
/**
|
|
* @param AbuseFilterViewExamine $page
|
|
* @param AbuseFilterChangesList $changesList
|
|
*/
|
|
public function __construct( AbuseFilterViewExamine $page, AbuseFilterChangesList $changesList ) {
|
|
parent::__construct();
|
|
$this->mChangesList = $changesList;
|
|
$this->mPage = $page;
|
|
}
|
|
|
|
/**
|
|
* @return array
|
|
*/
|
|
public function getQueryInfo() {
|
|
$dbr = wfGetDB( DB_REPLICA );
|
|
$conds = [];
|
|
|
|
if ( (string)$this->mPage->mSearchUser !== '' ) {
|
|
$conds[] = ActorMigration::newMigration()->getWhere(
|
|
$dbr, 'rc_user', User::newFromName( $this->mPage->mSearchUser, false )
|
|
)['conds'];
|
|
}
|
|
|
|
$startTS = strtotime( $this->mPage->mSearchPeriodStart );
|
|
if ( $startTS ) {
|
|
$conds[] = 'rc_timestamp>=' . $dbr->addQuotes( $dbr->timestamp( $startTS ) );
|
|
}
|
|
$endTS = strtotime( $this->mPage->mSearchPeriodEnd );
|
|
if ( $endTS ) {
|
|
$conds[] = 'rc_timestamp<=' . $dbr->addQuotes( $dbr->timestamp( $endTS ) );
|
|
}
|
|
|
|
$conds[] = $this->mPage->buildTestConditions( $dbr );
|
|
|
|
$rcQuery = RecentChange::getQueryInfo();
|
|
$info = [
|
|
'tables' => $rcQuery['tables'],
|
|
'fields' => $rcQuery['fields'],
|
|
'conds' => array_filter( $conds ),
|
|
'options' => [ 'ORDER BY' => 'rc_timestamp DESC' ],
|
|
'join_conds' => $rcQuery['joins'],
|
|
];
|
|
|
|
return $info;
|
|
}
|
|
|
|
/**
|
|
* @param stdClass $row
|
|
* @return string
|
|
*/
|
|
public function formatRow( $row ) {
|
|
$rc = RecentChange::newFromRow( $row );
|
|
$rc->counter = $this->mPage->mCounter++;
|
|
return $this->mChangesList->recentChangesLine( $rc, false );
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getIndexField() {
|
|
return 'rc_id';
|
|
}
|
|
|
|
/**
|
|
* @return Title
|
|
*/
|
|
public function getTitle() {
|
|
return $this->mPage->getTitle( 'examine' );
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getEmptyBody() {
|
|
return $this->msg( 'abusefilter-examine-noresults' )->parseAsBlock();
|
|
}
|
|
}
|