mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 18:19:38 +00:00
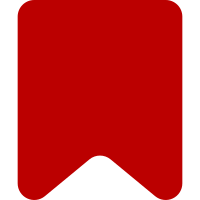
This commit doesn't change any permissions for anybody. It's the first step to achieve what the task asks for. Bug: T242821 Change-Id: I8060ca926e6769b11d470fe4037854cda496000d
90 lines
2.2 KiB
PHP
90 lines
2.2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Special;
|
|
|
|
use HtmlArmor;
|
|
use MediaWiki\Extension\AbuseFilter\AbuseFilterPermissionManager;
|
|
use SpecialPage;
|
|
use TitleValue;
|
|
use Xml;
|
|
|
|
/**
|
|
* Parent class for AbuseFilter special pages.
|
|
*/
|
|
abstract class AbuseFilterSpecialPage extends SpecialPage {
|
|
|
|
/** @var AbuseFilterPermissionManager */
|
|
protected $afPermissionManager;
|
|
|
|
/**
|
|
* @param string $name
|
|
* @param string $restriction
|
|
* @param AbuseFilterPermissionManager $afPermissionManager
|
|
*/
|
|
public function __construct(
|
|
$name,
|
|
$restriction,
|
|
AbuseFilterPermissionManager $afPermissionManager
|
|
) {
|
|
parent::__construct( $name, $restriction );
|
|
$this->afPermissionManager = $afPermissionManager;
|
|
}
|
|
|
|
/**
|
|
* Add topbar navigation links
|
|
*
|
|
* @param string $pageType
|
|
*/
|
|
protected function addNavigationLinks( $pageType ) {
|
|
$user = $this->getUser();
|
|
|
|
$linkDefs = [
|
|
'home' => 'AbuseFilter',
|
|
'recentchanges' => 'AbuseFilter/history',
|
|
'examine' => 'AbuseFilter/examine',
|
|
];
|
|
|
|
if ( $this->afPermissionManager->canViewAbuseLog( $user ) ) {
|
|
$linkDefs += [
|
|
'log' => 'AbuseLog'
|
|
];
|
|
}
|
|
|
|
if ( $this->afPermissionManager->canUseTestTools( $user ) ) {
|
|
$linkDefs += [
|
|
'test' => 'AbuseFilter/test',
|
|
'tools' => 'AbuseFilter/tools'
|
|
];
|
|
}
|
|
|
|
$links = [];
|
|
|
|
foreach ( $linkDefs as $name => $page ) {
|
|
// Give grep a chance to find the usages:
|
|
// abusefilter-topnav-home, abusefilter-topnav-recentchanges, abusefilter-topnav-test,
|
|
// abusefilter-topnav-log, abusefilter-topnav-tools, abusefilter-topnav-examine
|
|
$msgName = "abusefilter-topnav-$name";
|
|
|
|
$msg = $this->msg( $msgName )->parse();
|
|
|
|
if ( $name === $pageType ) {
|
|
$links[] = Xml::tags( 'strong', null, $msg );
|
|
} else {
|
|
$links[] = $this->getLinkRenderer()->makeLink(
|
|
new TitleValue( NS_SPECIAL, $page ),
|
|
new HtmlArmor( $msg )
|
|
);
|
|
}
|
|
}
|
|
|
|
$linkStr = $this->msg( 'parentheses' )
|
|
->rawParams( $this->getLanguage()->pipeList( $links ) )
|
|
->text();
|
|
$linkStr = $this->msg( 'abusefilter-topnav' )->parse() . " $linkStr";
|
|
|
|
$linkStr = Xml::tags( 'div', [ 'class' => 'mw-abusefilter-navigation' ], $linkStr );
|
|
|
|
$this->getOutput()->setSubtitle( $linkStr );
|
|
}
|
|
}
|