mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 10:15:24 +00:00
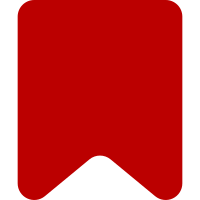
Thanks to this, we will be able to provide more information to consequences and watchers, which will open door for new features and possibly cleaner code. Change-Id: I7135509823ea84b2a2923d2c1831ce293b98a9f9
90 lines
2 KiB
PHP
90 lines
2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Parser;
|
|
|
|
class ParserStatus {
|
|
/** @var bool */
|
|
private $result;
|
|
/** @var bool */
|
|
private $warmCache;
|
|
/** @var AFPException|null */
|
|
private $excep;
|
|
/** @var UserVisibleWarning[] */
|
|
private $warnings;
|
|
|
|
/**
|
|
* @param bool $result A generic operation result
|
|
* @param bool $warmCache Whether we retrieved the AST from cache
|
|
* @param AFPException|null $excep An exception thrown while parsing, or null if it parsed correctly
|
|
* @param UserVisibleWarning[] $warnings
|
|
*/
|
|
public function __construct( bool $result, bool $warmCache, ?AFPException $excep, array $warnings ) {
|
|
$this->result = $result;
|
|
$this->warmCache = $warmCache;
|
|
$this->excep = $excep;
|
|
$this->warnings = $warnings;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getResult() : bool {
|
|
return $this->result;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getWarmCache() : bool {
|
|
return $this->warmCache;
|
|
}
|
|
|
|
/**
|
|
* @return AFPException|null
|
|
*/
|
|
public function getException() : ?AFPException {
|
|
return $this->excep;
|
|
}
|
|
|
|
/**
|
|
* @return UserVisibleWarning[]
|
|
*/
|
|
public function getWarnings() : array {
|
|
return $this->warnings;
|
|
}
|
|
|
|
/**
|
|
* Serialize data for edit stash
|
|
* @return array
|
|
*/
|
|
public function toArray() : array {
|
|
return [
|
|
'result' => $this->result,
|
|
'warmCache' => $this->warmCache,
|
|
'exception' => $this->excep ? $this->excep->toArray() : null,
|
|
'warnings' => array_map(
|
|
static function ( $warn ) {
|
|
return $warn->toArray();
|
|
},
|
|
$this->warnings
|
|
),
|
|
];
|
|
}
|
|
|
|
/**
|
|
* Deserialize data from edit stash
|
|
* @param array $value
|
|
* @return self
|
|
*/
|
|
public static function fromArray( array $value ) : self {
|
|
$excClass = $value['exception']['class'] ?? null;
|
|
return new self(
|
|
$value['result'],
|
|
$value['warmCache'],
|
|
$excClass !== null ? call_user_func( [ $excClass, 'fromArray' ], $value['exception'] ) : null,
|
|
array_map( [ UserVisibleWarning::class, 'fromArray' ], $value['warnings'] )
|
|
);
|
|
}
|
|
|
|
}
|