mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 10:15:24 +00:00
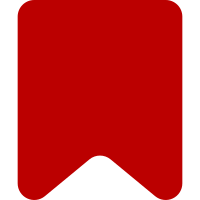
This is just a start; next step is adding a factory/store method to get/store these objects. And then use these value objects whenever applicable. Note: the actions-related code is still not fully implemented. This is going to happen as part of the FilterLookup. Change-Id: I5f33227887c035e301313bbe24d1c1fefb75bc6a
69 lines
1.2 KiB
PHP
69 lines
1.2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Filter;
|
|
|
|
/**
|
|
* (Mutable) value object that holds information about the last edit to a filter.
|
|
*/
|
|
class LastEditInfo {
|
|
/** @var int */
|
|
private $userID;
|
|
/** @var string */
|
|
private $userName;
|
|
/** @var string */
|
|
private $timestamp;
|
|
|
|
/**
|
|
* @param int $userID
|
|
* @param string $userName
|
|
* @param string $timestamp
|
|
*/
|
|
public function __construct( int $userID, string $userName, string $timestamp ) {
|
|
$this->userID = $userID;
|
|
$this->userName = $userName;
|
|
$this->timestamp = $timestamp;
|
|
}
|
|
|
|
/**
|
|
* @return int
|
|
*/
|
|
public function getUserID(): int {
|
|
return $this->userID;
|
|
}
|
|
|
|
/**
|
|
* @param int $id
|
|
*/
|
|
public function setUserID( int $id ): void {
|
|
$this->userID = $id;
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getUserName(): string {
|
|
return $this->userName;
|
|
}
|
|
|
|
/**
|
|
* @param string $name
|
|
*/
|
|
public function setUserName( string $name ): void {
|
|
$this->userName = $name;
|
|
}
|
|
|
|
/**
|
|
* @return string
|
|
*/
|
|
public function getTimestamp(): string {
|
|
return $this->timestamp;
|
|
}
|
|
|
|
/**
|
|
* @param string $timestamp
|
|
*/
|
|
public function setTimestamp( string $timestamp ): void {
|
|
$this->timestamp = $timestamp;
|
|
}
|
|
}
|