mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-15 10:15:24 +00:00
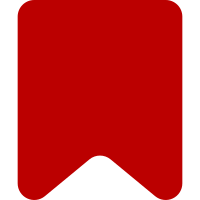
This replaces the previous pattern of callers having to use RevisionLookup if the result was 'implicit'. Also, in some cases where we were just hiding things if the visibility was !== true, properly handle the implicit case by using the new method. Make the new method return string constants rather than bool|string. The new method also fixes some potential info leaks which happened when the row was hidden, the user could view suppressed AbuseLog entries, but the associated revision was also deleted and the user couldn't see it (this shouldn't be relevant for WMF wikis since AF deletion is oversight-level). Also add a bunch of tests for the various cases to ensure we don't regress again. Bug: T261532 Change-Id: I929f865acf5d207b739cb3af043f70cb59243ee0
72 lines
2.1 KiB
PHP
72 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter;
|
|
|
|
use MediaWiki\Extension\AbuseFilter\Variables\VariableHolder;
|
|
use RequestContext;
|
|
use Status;
|
|
use Title;
|
|
use User;
|
|
|
|
/**
|
|
* This class contains most of the business logic of AbuseFilter. It consists of
|
|
* static functions for generic use (mostly utility functions).
|
|
*/
|
|
class AbuseFilter {
|
|
|
|
/**
|
|
* @deprecated
|
|
* @todo Phase out
|
|
*/
|
|
public const HISTORY_MAPPINGS = [
|
|
'af_pattern' => 'afh_pattern',
|
|
'af_user' => 'afh_user',
|
|
'af_user_text' => 'afh_user_text',
|
|
'af_timestamp' => 'afh_timestamp',
|
|
'af_comments' => 'afh_comments',
|
|
'af_public_comments' => 'afh_public_comments',
|
|
'af_deleted' => 'afh_deleted',
|
|
'af_id' => 'afh_filter',
|
|
'af_group' => 'afh_group',
|
|
];
|
|
|
|
/**
|
|
* Returns an associative array of filters which were tripped
|
|
*
|
|
* @param VariableHolder $vars
|
|
* @param Title $title
|
|
* @return bool[] Map of (filter ID => bool)
|
|
* @deprecated Since 1.34 This was meant to be internal!
|
|
* @codeCoverageIgnore Deprecated method
|
|
*/
|
|
public static function checkAllFilters(
|
|
VariableHolder $vars,
|
|
Title $title
|
|
) {
|
|
$user = RequestContext::getMain()->getUser();
|
|
$runnerFactory = AbuseFilterServices::getFilterRunnerFactory();
|
|
$runner = $runnerFactory->newRunner( $user, $title, $vars, 'default' );
|
|
return $runner->checkAllFilters();
|
|
}
|
|
|
|
/**
|
|
* @param VariableHolder $vars
|
|
* @param Title $title
|
|
* @param string $group The filter's group (as defined in $wgAbuseFilterValidGroups)
|
|
* @param User $user The user performing the action
|
|
* @return Status
|
|
* @deprecated Since 1.34 Get a FilterRunner instance and call run() on that, if you really need to.
|
|
* Or consider resolving the problem at its root, because you shouldn't need to call this manually.
|
|
* @codeCoverageIgnore Deprecated method
|
|
*/
|
|
public static function filterAction(
|
|
VariableHolder $vars, Title $title, $group, User $user
|
|
) {
|
|
$runnerFactory = AbuseFilterServices::getFilterRunnerFactory();
|
|
$runner = $runnerFactory->newRunner( $user, $title, $vars, $group );
|
|
return $runner->run();
|
|
}
|
|
}
|
|
|
|
class_alias( AbuseFilter::class, 'AbuseFilter' );
|