mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-23 21:53:35 +00:00
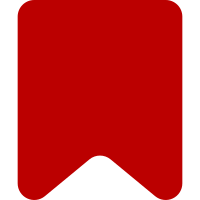
- Rename `$hidden` to `$privacyLevel` in Flags::__construct for consistency with other places. - Rename `shouldProtectFilter` and simplify its return value to always be an array, since that's how it's currently used. Rename a variable that is assigned the return value of this method. - Add a missing message key to a list of dynamic message keys. - Rename a property from 'hidden' to 'privacy' in FilterStoreTest for consistency. Add a test for removing the protected flag. - Update old comment referencing `filterHidden`; the method was removed in I40b8c8452d9df. - Use ISQLPlatform::bitAnd() instead of manual SQL in AbuseFilterHistoryPager. - Update mysterious reference to "formatRow" in SpecialAbuseLog. - Update other references to the very same method in two other places, this time credited as "SpecialAbuseLog". - Add type hints to a few methods; this not only helps with type safety, but it also allows PHPUnit to automatically use the proper type in mocks. Change-Id: Ib0167d993b761271c1e5311808435a616b6576fe
126 lines
2.4 KiB
PHP
126 lines
2.4 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter\Filter;
|
|
|
|
/**
|
|
* (Mutable) value object to represent flags that can be *manually* set on a filter.
|
|
*/
|
|
class Flags {
|
|
/** @var bool */
|
|
private $enabled;
|
|
/** @var bool */
|
|
private $deleted;
|
|
/** @var bool */
|
|
private $hidden;
|
|
/** @var bool */
|
|
private $protected;
|
|
/** @var int */
|
|
private $privacyLevel;
|
|
/** @var bool */
|
|
private $global;
|
|
|
|
public const FILTER_PUBLIC = 0b00;
|
|
public const FILTER_HIDDEN = 0b01;
|
|
public const FILTER_USES_PROTECTED_VARS = 0b10;
|
|
|
|
/**
|
|
* @param bool $enabled
|
|
* @param bool $deleted
|
|
* @param int $privacyLevel
|
|
* @param bool $global
|
|
*/
|
|
public function __construct( bool $enabled, bool $deleted, int $privacyLevel, bool $global ) {
|
|
$this->enabled = $enabled;
|
|
$this->deleted = $deleted;
|
|
$this->hidden = (bool)( self::FILTER_HIDDEN & $privacyLevel );
|
|
$this->protected = (bool)( self::FILTER_USES_PROTECTED_VARS & $privacyLevel );
|
|
$this->privacyLevel = $privacyLevel;
|
|
$this->global = $global;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getEnabled(): bool {
|
|
return $this->enabled;
|
|
}
|
|
|
|
/**
|
|
* @param bool $enabled
|
|
*/
|
|
public function setEnabled( bool $enabled ): void {
|
|
$this->enabled = $enabled;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getDeleted(): bool {
|
|
return $this->deleted;
|
|
}
|
|
|
|
/**
|
|
* @param bool $deleted
|
|
*/
|
|
public function setDeleted( bool $deleted ): void {
|
|
$this->deleted = $deleted;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getHidden(): bool {
|
|
return $this->hidden;
|
|
}
|
|
|
|
/**
|
|
* @param bool $hidden
|
|
*/
|
|
public function setHidden( bool $hidden ): void {
|
|
$this->hidden = $hidden;
|
|
$this->updatePrivacyLevel();
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getProtected(): bool {
|
|
return $this->protected;
|
|
}
|
|
|
|
/**
|
|
* @param bool $protected
|
|
*/
|
|
public function setProtected( bool $protected ): void {
|
|
$this->protected = $protected;
|
|
$this->updatePrivacyLevel();
|
|
}
|
|
|
|
private function updatePrivacyLevel() {
|
|
$hidden = $this->hidden ? self::FILTER_HIDDEN : 0;
|
|
$protected = $this->protected ? self::FILTER_USES_PROTECTED_VARS : 0;
|
|
$this->privacyLevel = $hidden | $protected;
|
|
}
|
|
|
|
/**
|
|
* @return int
|
|
*/
|
|
public function getPrivacyLevel(): int {
|
|
return $this->privacyLevel;
|
|
}
|
|
|
|
/**
|
|
* @return bool
|
|
*/
|
|
public function getGlobal(): bool {
|
|
return $this->global;
|
|
}
|
|
|
|
/**
|
|
* @param bool $global
|
|
*/
|
|
public function setGlobal( bool $global ): void {
|
|
$this->global = $global;
|
|
}
|
|
}
|