mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/AbuseFilter.git
synced 2024-11-13 17:27:20 +00:00
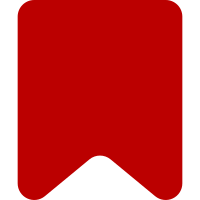
Protected variables will cause the filter using them to become protected as well. `af_hidden` can be used to track this flag, as it is a TINYINT and can be converted into a bitmask with no schema changes. This is not a backwards-compatible change, as now all checks must check the `hidden` flag specifically or otherwise will be cast to true if any flag is set. To support this change: - "hidden" is considered a flag set in the `af_hidden`. This is a change in concept with no need for updates to the column values, as there is currently only one flag in the bitmask. - `Flag`s store the bitmask as well as the state of single flags and can return either. - Any checks against the `af_hidden` value no longer check a boolean value and instead now check the `hidden` flag value. Bug: T363906 Change-Id: I358205cb1119cf1e4004892c37e36e0c0a864f37
73 lines
2.1 KiB
PHP
73 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\AbuseFilter;
|
|
|
|
use MediaWiki\Extension\AbuseFilter\Consequences\ConsequencesRegistry;
|
|
use MediaWiki\Extension\AbuseFilter\Filter\Filter;
|
|
|
|
/**
|
|
* This service allows comparing two versions of a filter.
|
|
* @todo We might want to expand this to cover the use case of ViewDiff
|
|
* @internal
|
|
*/
|
|
class FilterCompare {
|
|
public const SERVICE_NAME = 'AbuseFilterFilterCompare';
|
|
|
|
/** @var ConsequencesRegistry */
|
|
private $consequencesRegistry;
|
|
|
|
/**
|
|
* @param ConsequencesRegistry $consequencesRegistry
|
|
*/
|
|
public function __construct( ConsequencesRegistry $consequencesRegistry ) {
|
|
$this->consequencesRegistry = $consequencesRegistry;
|
|
}
|
|
|
|
/**
|
|
* @param Filter $firstFilter
|
|
* @param Filter $secondFilter
|
|
* @return array Fields that are different
|
|
*/
|
|
public function compareVersions( Filter $firstFilter, Filter $secondFilter ): array {
|
|
// TODO: Avoid DB references here, re-add when saving the filter
|
|
$methods = [
|
|
'af_public_comments' => 'getName',
|
|
'af_pattern' => 'getRules',
|
|
'af_comments' => 'getComments',
|
|
'af_deleted' => 'isDeleted',
|
|
'af_enabled' => 'isEnabled',
|
|
'af_hidden' => 'getPrivacyLevel',
|
|
'af_global' => 'isGlobal',
|
|
'af_group' => 'getGroup',
|
|
];
|
|
|
|
$differences = [];
|
|
|
|
foreach ( $methods as $field => $method ) {
|
|
if ( $firstFilter->$method() !== $secondFilter->$method() ) {
|
|
$differences[] = $field;
|
|
}
|
|
}
|
|
|
|
$firstActions = $firstFilter->getActions();
|
|
$secondActions = $secondFilter->getActions();
|
|
foreach ( $this->consequencesRegistry->getAllEnabledActionNames() as $action ) {
|
|
if ( !isset( $firstActions[$action] ) && !isset( $secondActions[$action] ) ) {
|
|
// They're both unset
|
|
} elseif ( isset( $firstActions[$action] ) && isset( $secondActions[$action] ) ) {
|
|
// They're both set. Double check needed, e.g. per T180194
|
|
if ( array_diff( $firstActions[$action], $secondActions[$action] ) ||
|
|
array_diff( $secondActions[$action], $firstActions[$action] ) ) {
|
|
// Different parameters
|
|
$differences[] = 'actions';
|
|
}
|
|
} else {
|
|
// One's unset, one's set.
|
|
$differences[] = 'actions';
|
|
}
|
|
}
|
|
|
|
return array_unique( $differences );
|
|
}
|
|
}
|